Explore how Jenkins versions have shaped modern CI/CD practices. This comprehensive guide traces the …
9 Jenkins Hacks That Will Make Your Life Easier - DevOps 9 Jenkins Hacks That Will Make Your Life Easier - DevOps
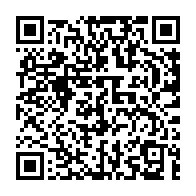
Summary
Introduction to Jenkins Hacks
Understanding how to optimize Jenkins is crucial in today’s fast-paced development environment. These nine carefully selected Jenkins hacks represent years of collective experience from DevOps engineers and system administrators who have found ways to enhance Jenkins’ capabilities beyond its default configuration. Think of these hacks as power tools in your toolbox – while Jenkins works fine with basic tools, these advanced techniques will help you build more efficiently and reliably.
Before we dive into the specific hacks, it’s important to understand why they matter. In modern software development, the difference between a good CI/CD pipeline and a great one often comes down to how well you’ve optimized your Jenkins setup. These hacks address common pain points like slow build times, security vulnerabilities, and maintenance overhead.
Expand your knowledge with Comprehensive Guide to Jenkins Versions: Implementation, Best Practices, and Real-world Examples
Hack #1: Mastering Jenkins Pipeline Configuration
The foundation of an efficient Jenkins setup lies in how you configure your pipelines. This hack focuses on leveraging declarative pipeline syntax to create more maintainable and readable configurations. Think of your pipeline configuration as the blueprint for your software delivery process – the clearer and more structured it is, the easier it will be to build and maintain your project.
Let’s examine a comprehensive pipeline configuration that incorporates several best practices:
pipeline {
agent any
// Define tools we'll use throughout the pipeline
tools {
maven 'Maven-3.8.1'
jdk 'JDK-11'
}
// Pipeline-wide environment variables
environment {
ARTIFACT_NAME = 'my-application'
VERSION = sh(script: 'git describe --tags', returnStdout: true).trim()
BUILD_TIMESTAMP = sh(script: 'date "+%Y%m%d_%H%M%S"', returnStdout: true).trim()
}
// Pipeline options
options {
timeout(time: 1, unit: 'HOURS')
buildDiscarder(logRotator(numToKeepStr: '10'))
timestamps()
disableConcurrentBuilds()
}
stages {
stage('Preparation') {
steps {
script {
// Record build start time for metrics
env.BUILD_START_TIME = System.currentTimeMillis()
// Validate environment
sh '''
echo "Validating build environment..."
mvn --version
java -version
git --version
'''
}
}
}
stage('Build') {
steps {
script {
try {
// Run the build with detailed logging
sh """
echo "Building ${ARTIFACT_NAME} version ${VERSION}..."
mvn clean package \
-Drevision=${VERSION} \
-DskipTests \
-Dfile.encoding=UTF-8 \
-Dmaven.test.failure.ignore=false
"""
// Archive the artifacts
archiveArtifacts artifacts: 'target/*.jar', fingerprint: true
} catch (Exception e) {
currentBuild.result = 'FAILURE'
error("Build failed: ${e.message}")
}
}
}
}
stage('Test') {
steps {
script {
try {
// Run tests with coverage
sh """
echo "Running tests for ${ARTIFACT_NAME}..."
mvn test jacoco:report
"""
} catch (Exception e) {
currentBuild.result = 'UNSTABLE'
error("Tests failed: ${e.message}")
}
}
}
post {
always {
// Publish test and coverage results
junit '**/target/surefire-reports/*.xml'
jacoco execPattern: 'target/jacoco.exec'
// Calculate test metrics
script {
def testDuration = System.currentTimeMillis() - env.BUILD_START_TIME.toLong()
echo "Tests completed in ${testDuration}ms"
}
}
}
}
}
post {
success {
script {
// Calculate total build time
def totalDuration = System.currentTimeMillis() - env.BUILD_START_TIME.toLong()
echo "Build completed successfully in ${totalDuration}ms"
// Notify team of success
emailext (
subject: "Build Successful: ${env.JOB_NAME} #${env.BUILD_NUMBER}",
body: """
Build completed successfully!
Job: ${env.JOB_NAME}
Build Number: ${env.BUILD_NUMBER}
Duration: ${totalDuration}ms
Artifact: ${ARTIFACT_NAME}
Version: ${VERSION}
Check console output at: ${env.BUILD_URL}
""",
recipientProviders: [[$class: 'DevelopersRecipientProvider']]
)
}
}
failure {
script {
// Notify team of failure
emailext (
subject: "Build Failed: ${env.JOB_NAME} #${env.BUILD_NUMBER}",
body: """
Build failed!
Job: ${env.JOB_NAME}
Build Number: ${env.BUILD_NUMBER}
Error: ${currentBuild.description ?: 'Unknown error'}
Check console output at: ${env.BUILD_URL}
""",
recipientProviders: [[$class: 'DevelopersRecipientProvider']],
attachLog: true
)
}
}
always {
// Clean workspace
cleanWs()
}
}
}
This pipeline configuration demonstrates several important concepts:
Environment Setup: The pipeline defines tools and environment variables at the beginning, making them available throughout the build process.
Build Options: Important options like timeout and build retention policies are defined clearly.
Stage Organization: The pipeline is organized into logical stages (Preparation, Build, Test) with clear responsibilities.
Error Handling: Each stage includes proper error handling and reporting.
Post-Build Actions: The pipeline includes comprehensive post-build actions for both success and failure scenarios.
Metrics Collection: The pipeline tracks build times and other metrics for performance monitoring.
Deepen your understanding in Jenkinsfile with envsubst: Simplifying CI/CD Pipeline Configuration
Hack #2: Pre-Installing Essential Jenkins Plugins
Plugin management represents a critical aspect of Jenkins administration that many teams struggle with. Think of Jenkins plugins as extensions to your toolbox – while you don’t want to overcrowd it, having the right tools ready when you need them is crucial. This hack focuses on automating plugin installation and management to ensure consistency across your Jenkins environments.
Here’s a comprehensive approach to plugin management:
import jenkins.model.*
import java.util.logging.Logger
import hudson.model.UpdateSite
import hudson.PluginWrapper
def logger = Logger.getLogger("")
def jenkins = Jenkins.getInstance()
def pm = jenkins.getPluginManager()
def uc = jenkins.getUpdateCenter()
// Essential plugins for a robust CI/CD setup
def requiredPlugins = [
// Core functionality
'workflow-aggregator', // Pipeline
'git', // Git integration
'configuration-as-code', // Configuration as code
// Build and test
'maven-plugin', // Maven integration
'junit', // Test results
'jacoco', // Code coverage
// Security
'credentials', // Credentials management
'role-strategy', // Role-based access control
// Monitoring
'prometheus', // Metrics collection
'performance', // Performance testing
// Notifications
'email-ext', // Extended email
'slack', // Slack integration
// UI and visualization
'blueocean', // Modern UI
'dashboard-view' // Custom dashboards
]
// Update the update center
logger.info("Updating Jenkins Update Center")
uc.updateAllSites()
def installPlugin = { pluginId ->
def plugin = pm.getPlugin(pluginId)
if (!plugin) {
logger.info("Installing ${pluginId}")
def deployment = uc.getPlugin(pluginId).deploy(true)
deployment.get()
} else if (plugin.hasUpdate()) {
logger.info("Updating ${pluginId}")
plugin.doUpgrade()
} else {
logger.info("${pluginId} already installed and up to date")
}
}
// Install/update required plugins
requiredPlugins.each { pluginId ->
try {
installPlugin(pluginId)
} catch (Exception e) {
logger.warning("Failed to install ${pluginId}: ${e.message}")
}
}
// Check for plugin dependencies
def checkDependencies = { plugin ->
plugin.getDependencies().each { dependency ->
def dependencyId = dependency.shortName
if (!pm.getPlugin(dependencyId)) {
logger.info("Installing dependency: ${dependencyId}")
installPlugin(dependencyId)
}
}
}
// Verify all plugins are correctly installed
pm.plugins.each { plugin ->
checkDependencies(plugin)
}
// Save changes and check if restart is required
if (pm.needsRestart()) {
logger.info("Jenkins needs to be restarted")
jenkins.restart()
} else {
logger.info("No restart required")
}
This script demonstrates several important plugin management concepts:
Plugin Selection: The script defines a curated list of essential plugins categorized by functionality.
Dependency Management: It automatically handles plugin dependencies to ensure all required components are installed.
Version Control: The script checks for updates and manages plugin versions.
Error Handling: Robust error handling ensures the script continues even if individual plugin installations fail.
Restart Management: The script intelligently handles Jenkins restarts when required.
Explore this further in Comprehensive Guide to Jenkins Versions: Implementation, Best Practices, and Real-world Examples
Hack #3: Custom Port Configuration for Enhanced Security
Changing Jenkins’ default port is a simple yet effective security measure. Think of it as changing the lock on your front door – while it’s not the only security measure you need, it’s an important basic step. This hack explores how to implement custom port configuration properly while maintaining accessibility.
Here’s a comprehensive approach to port configuration:
Discover related concepts in Security Considerations When Using envsubst: Protecting Your CI/CD Pipeline
#!/bin/bash
# Configuration script for Jenkins port
# Define variables
JENKINS_PORT=8083
JENKINS_CONFIG="/etc/default/jenkins"
JENKINS_XML="/etc/init.d/jenkins"
SYSTEMD_SERVICE="/etc/systemd/system/jenkins.service"
# Function to backup configuration files
backup_configs() {
local timestamp=$(date +%Y%m%d_%H%M%S)
if [ -f "$JENKINS_CONFIG" ]; then
cp "$JENKINS_CONFIG" "${JENKINS_CONFIG}.backup_${timestamp}"
echo "Backed up $JENKINS_CONFIG"
fi
if [ -f "$JENKINS_XML" ]; then
cp "$JENKINS_XML" "${JENKINS_XML}.backup_${timestamp}"
echo "Backed up $JENKINS_XML"
fi
if [ -f "$SYSTEMD_SERVICE" ]; then
cp "$SYSTEMD_SERVICE" "${SYSTEMD_SERVICE}.backup_${timestamp}"
echo "Backed up $SYSTEMD_SERVICE"
fi
}
# Function to update Jenkins configuration
update_jenkins_config() {
# Update /etc/default/jenkins
if [ -f "$JENKINS_CONFIG" ]; then
# Remove existing HTTP port configuration
sed -i '/^HTTP_PORT/d' "$JENKINS_CONFIG"
# Add new HTTP port configuration
echo "HTTP_PORT=$JENKINS_PORT" >> "$JENKINS_CONFIG"
echo "Updated $JENKINS_CONFIG"
fi
# Update systemd service if it exists
if [ -f "$SYSTEMD_SERVICE" ]; then
sed -i "s/--httpPort=[0-9]*/--httpPort=$JENKINS_PORT/" "$SYSTEMD_SERVICE"
echo "Updated $SYSTEMD_SERVICE"
# Reload systemd configuration
systemctl daemon-reload
fi
}
# Function to verify port availability
check_port() {
if netstat -tuln | grep ":$JENKINS_PORT " > /dev/null; then
echo "Warning: Port $JENKINS_PORT is already in use"
exit 1
fi
}
# Function to update firewall rules
update_firewall() {
# For UFW (Uncomplicated Firewall)
if command -v ufw >/dev/null 2>&1; then
ufw allow $JENKINS_PORT/tcp
echo "Updated UFW rules"
fi
# For firewalld
if command -v firewall-cmd >/dev/null 2>&1; then
firewall-cmd --permanent --add-port=$JENKINS_PORT/tcp
firewall-cmd --reload
echo "Updated firewalld rules"
fi
}
# Main execution
echo "Starting Jenkins port configuration..."
# Check if running as root
if [ "$EUID" -ne 0 ]; then
echo "Please run as root"
exit 1
fi
# Execute configuration steps
check_port
backup_configs
update_jenkins_config
update_firewall
# Restart Jenkins
echo "Restarting Jenkins..."
systemctl restart jenkins
# Verify Jenkins startup
echo "Waiting for Jenkins to start..."
timeout 60 bash -c 'until curl -s http://localhost:$JENKINS_PORT > /dev/null; do sleep 5; done'
if [ $? -eq 0 ]; then
echo "Jenkins successfully started on port $JENKINS_PORT"
else
echo "Warning: Jenkins startup verification timed out"
fi
echo "Configuration complete. Please verify Jenkins is accessible at http://localhost:$JENKINS_PORT"
Hack #4: Implementing Shared Libraries
Shared libraries represent one of the most powerful features in Jenkins, yet they’re often underutilized. Think of shared libraries as creating your own toolkit of reusable functions that can be shared across all your pipelines. This approach not only reduces code duplication but also ensures consistency across your organization’s CI/CD processes.
Let’s explore a comprehensive shared library implementation:
// vars/deployToEnvironment.groovy
def call(Map config) {
// Input validation with helpful error messages
if (!config.environment) {
error "Environment must be specified. Valid options are: 'dev', 'staging', 'prod'"
}
if (!config.appName) {
error "Application name must be specified"
}
// Default configuration with override options
def defaultConfig = [
timeout: 30,
healthCheckRetries: 5,
healthCheckDelay: 10,
notifyOnSuccess: true,
notifyChannel: '#deployments'
]
// Merge provided config with defaults
config = defaultConfig + config
pipeline {
agent any
environment {
DEPLOY_ENV = config.environment
APP_NAME = config.appName
VERSION = sh(script: 'git describe --tags', returnStdout: true).trim()
}
stages {
stage('Environment Validation') {
steps {
script {
// Validate environment-specific prerequisites
validateEnvironment(config.environment)
// Load environment-specific configuration
def envConfig = loadEnvironmentConfig(config.environment)
env.DEPLOY_CONFIG = envConfig
}
}
}
stage('Deploy') {
steps {
timeout(time: config.timeout, unit: 'MINUTES') {
script {
try {
// Execute deployment
performDeploy(config)
// Verify deployment
verifyDeployment(config)
// Record deployment metrics
recordMetrics(config)
} catch (Exception e) {
handleDeploymentFailure(e, config)
throw e
}
}
}
}
}
}
post {
success {
script {
if (config.notifyOnSuccess) {
notifyDeploymentStatus('success', config)
}
}
}
failure {
script {
notifyDeploymentStatus('failure', config)
}
}
}
}
}
// Helper functions
def validateEnvironment(String environment) {
def validEnvironments = ['dev', 'staging', 'prod']
if (!validEnvironments.contains(environment)) {
error "Invalid environment: ${environment}. Must be one of: ${validEnvironments}"
}
// Environment-specific validation
switch(environment) {
case 'prod':
validateProductionDeploy()
break
case 'staging':
validateStagingDeploy()
break
default:
validateDevDeploy()
}
}
def performDeploy(Map config) {
echo "Starting deployment to ${config.environment}"
// Environment-specific deployment logic
switch(config.environment) {
case 'prod':
deployToProduction(config)
break
case 'staging':
deployToStaging(config)
break
default:
deployToDevelopment(config)
}
}
def verifyDeployment(Map config) {
def retries = config.healthCheckRetries
def delay = config.healthCheckDelay
echo "Verifying deployment with ${retries} retries, ${delay}s delay"
for (int i = 0; i < retries; i++) {
try {
// Perform health check
def status = sh(
script: "curl -sf http://${config.appName}.${config.environment}/health",
returnStatus: true
)
if (status == 0) {
echo "Deployment verified successfully"
return
}
} catch (Exception e) {
echo "Health check attempt ${i + 1} failed: ${e.message}"
}
sleep delay
}
error "Deployment verification failed after ${retries} attempts"
}
def notifyDeploymentStatus(String status, Map config) {
def color = status == 'success' ? 'good' : 'danger'
def message = """
Deployment ${status}: ${config.appName}
Environment: ${config.environment}
Version: ${env.VERSION}
Build: ${env.BUILD_URL}
""".stripIndent()
slackSend(
channel: config.notifyChannel,
color: color,
message: message
)
}
This shared library implementation demonstrates several important concepts:
Configuration Management: The library provides sensible defaults while allowing overrides, making it flexible yet safe to use.
Environment Validation: It includes robust environment validation to prevent deployment mistakes.
Error Handling: Comprehensive error handling ensures failures are caught and properly reported.
Deployment Verification: The library includes built-in health checks and verification.
Notifications: Integrated notification system keeps teams informed of deployment status.
To use this shared library in your Jenkinsfile:
Uncover more details in Master Ansible: Tips, Tricks, and Hacks for Secure and Efficient Automation
@Library('my-shared-lib') _
deployToEnvironment([
appName: 'my-application',
environment: 'staging',
timeout: 45,
healthCheckRetries: 10,
notifyChannel: '#team-deployments'
])
Hack #5: Advanced Environment Variable Management
Environment variables in Jenkins are more powerful than most users realize. Proper environment variable management can make your pipelines more secure, flexible, and maintainable. Let’s explore advanced techniques for handling environment variables:
Journey deeper into this topic with Master Ansible: Tips, Tricks, and Hacks for Secure and Efficient Automation
pipeline {
agent any
// Dynamic environment variable generation
environment {
// Generate build-specific variables
BUILD_ID = "${env.BUILD_NUMBER}-${env.GIT_COMMIT[0..7]}"
TIMESTAMP = sh(script: 'date "+%Y%m%d_%H%M%S"', returnStdout: true).trim()
// Load environment-specific variables
DEPLOY_ENV = determineEnvironment()
// Credential management
AWS_CREDENTIALS = credentials('aws-credentials')
DATABASE_URL = credentials('database-url')
// Configuration variables
CONFIG = loadConfiguration()
}
stages {
stage('Environment Setup') {
steps {
script {
// Display environment information
echo "Build ID: ${env.BUILD_ID}"
echo "Environment: ${env.DEPLOY_ENV}"
// Validate environment variables
validateEnvironmentVariables()
// Load additional environment-specific variables
loadEnvironmentSpecificVars()
}
}
}
stage('Deploy') {
steps {
script {
withCredentials([
string(credentialsId: 'api-key', variable: 'API_KEY'),
usernamePassword(
credentialsId: 'db-credentials',
usernameVariable: 'DB_USER',
passwordVariable: 'DB_PASS'
)
]) {
// Use credentials securely
sh """
./deploy.sh \
--env ${DEPLOY_ENV} \
--api-key ${API_KEY} \
--db-url ${DATABASE_URL} \
--config ${CONFIG}
"""
}
}
}
}
}
}
// Helper function to determine environment
def determineEnvironment() {
if (env.BRANCH_NAME == 'main') {
return 'production'
} else if (env.BRANCH_NAME == 'staging') {
return 'staging'
}
return 'development'
}
// Helper function to load configuration
def loadConfiguration() {
def config = [:]
// Load base configuration
def baseConfig = readJSON file: 'config/base.json'
config.putAll(baseConfig)
// Load environment-specific configuration
def envConfig = readJSON file: "config/${DEPLOY_ENV}.json"
config.putAll(envConfig)
return writeJSON(json: config, returnText: true)
}
// Helper function to validate environment variables
def validateEnvironmentVariables() {
def requiredVars = [
'BUILD_ID',
'DEPLOY_ENV',
'AWS_CREDENTIALS',
'DATABASE_URL'
]
requiredVars.each { var ->
if (!env[var]) {
error "Required environment variable ${var} is not set"
}
}
}
// Helper function to load environment-specific variables
def loadEnvironmentSpecificVars() {
def envFile = "config/env/${DEPLOY_ENV}.env"
if (fileExists(envFile)) {
def envVars = readFile(envFile).split('\n')
envVars.each { line ->
if (line && !line.startsWith('#')) {
def (key, value) = line.split('=', 2)
env[key.trim()] = value.trim()
}
}
}
}
Hack #6: Advanced Security Configuration
Security in Jenkins requires a comprehensive approach that goes beyond basic authentication. Think of Jenkins security like protecting a valuable asset - you need multiple layers of defense. Let’s explore how to implement advanced security measures that protect your CI/CD pipeline while maintaining usability.
// security.groovy - Jenkins initialization script
import jenkins.model.*
import hudson.security.*
import org.jenkinsci.plugins.matrixauth.*
import com.cloudbees.plugins.credentials.*
import com.cloudbees.plugins.credentials.domains.*
import org.jenkinsci.plugins.workflow.job.WorkflowJob
def jenkins = Jenkins.getInstance()
// Configure Global Security
void configureGlobalSecurity() {
def strategy = new FullControlOnceLoggedInAuthorizationStrategy()
strategy.setAllowAnonymousRead(false)
jenkins.setAuthorizationStrategy(strategy)
def realm = new HudsonPrivateSecurityRealm(false)
jenkins.setSecurityRealm(realm)
// Configure CSRF protection
jenkins.setCrumbIssuer(new DefaultCrumbIssuer(true))
}
// Configure Matrix-based Security
void configureMatrixSecurity() {
def strategy = new GlobalMatrixAuthorizationStrategy()
// Define roles and permissions
def roles = [
'admin': [
'hudson.model.Hudson.Administer',
'hudson.model.Hudson.Read',
'hudson.model.Item.Build',
'hudson.model.Item.Cancel',
'hudson.model.Item.Configure',
'hudson.model.Item.Create',
'hudson.model.Item.Delete',
'hudson.model.Item.Read'
],
'developer': [
'hudson.model.Hudson.Read',
'hudson.model.Item.Build',
'hudson.model.Item.Cancel',
'hudson.model.Item.Read'
],
'viewer': [
'hudson.model.Hudson.Read',
'hudson.model.Item.Read'
]
]
// Apply permissions for each role
roles.each { role, permissions ->
permissions.each { permission ->
strategy.add(permission, "${role}")
}
}
jenkins.setAuthorizationStrategy(strategy)
}
// Configure Agent Security
void configureAgentSecurity() {
jenkins.getInjector().getInstance(AdminWhitelistRule.class)
.setMasterKillSwitch(false)
// Configure agent protocols
Set<String> agentProtocols = ['JNLP4-connect']
jenkins.setAgentProtocols(agentProtocols)
// Configure agent security options
jenkins.getExtensionList(GlobalConfiguration.class)
.get(GlobalSecurityConfiguration.class).with {
it.getAgentProtocol().setEnabled(false)
it.getUsageStatisticsEnabled().setEnabled(false)
}
}
// Configure Script Security
void configureScriptSecurity() {
def scriptApproval = jenkins.getExtensionList(
'org.jenkinsci.plugins.scriptsecurity.scripts.ScriptApproval'
)[0]
// Approve specific signatures for trusted scripts
def approvedSignatures = [
'method java.lang.String trim',
'method java.lang.String split java.lang.String',
'new java.lang.StringBuilder',
'method java.lang.StringBuilder append java.lang.String',
'method java.lang.StringBuilder toString'
]
approvedSignatures.each { signature ->
scriptApproval.approveSignature(signature)
}
}
// Implement secure pipeline practices
void configurePipelineSecurity() {
// Create a secure pipeline template
def securePipeline = '''
pipeline {
agent any
options {
// Prevent concurrent builds
disableConcurrentBuilds()
// Timeout to prevent hanging builds
timeout(time: 1, unit: 'HOURS')
}
stages {
stage('Security Scan') {
steps {
script {
// Perform security scanning
def scanResult = sh(
script: '''
./security-scan.sh \
--severity high \
--fail-on-findings
''',
returnStatus: true
)
if (scanResult != 0) {
error "Security scan failed"
}
}
}
}
stage('Deploy') {
steps {
withCredentials([
usernamePassword(
credentialsId: 'deploy-credentials',
usernameVariable: 'DEPLOY_USER',
passwordVariable: 'DEPLOY_PASS'
)
]) {
// Secure deployment steps
sh '''
./deploy.sh \
--user ${DEPLOY_USER} \
--pass ${DEPLOY_PASS} \
--secure-mode
'''
}
}
}
}
post {
always {
// Clean workspace to prevent sensitive data leaks
cleanWs()
}
}
}
'''
// Create a secure pipeline job
def pipelineJob = jenkins.createProject(WorkflowJob, 'secure-pipeline-template')
pipelineJob.setDefinition(new CpsFlowDefinition(securePipeline, true))
}
// Main execution
try {
// Execute security configurations
configureGlobalSecurity()
configureMatrixSecurity()
configureAgentSecurity()
configureScriptSecurity()
configurePipelineSecurity()
jenkins.save()
println "Security configuration completed successfully"
} catch (Exception e) {
println "Error configuring security: ${e.message}"
e.printStackTrace()
throw e
}
This security configuration demonstrates several important concepts:
Multi-layered Security: The configuration implements multiple security layers, including authentication, authorization, and CSRF protection.
Role-based Access Control: It defines different roles (admin, developer, viewer) with appropriate permissions for each.
Agent Security: Configures secure agent protocols and communication.
Script Security: Implements script approval mechanisms to prevent unauthorized code execution.
Secure Pipeline Practices: Provides a template for creating secure pipelines with proper credential handling and workspace cleanup.
Enrich your learning with Dynamic Configuration Management with envsubst and Jinja2: Simplifying Deployments
Hack #7: Advanced Pipeline Monitoring and Metrics
Understanding how your pipelines perform is crucial for maintaining and improving your CI/CD process. Let’s explore how to implement comprehensive monitoring and metrics collection:
Gain comprehensive insights from Advanced Guide to Using the vmstat Command in Linux
// monitoring.groovy
pipeline {
agent any
options {
timestamps()
timeout(time: 1, unit: 'HOURS')
}
environment {
// Metric collection configuration
METRIC_PREFIX = 'jenkins.pipeline'
STATSD_HOST = 'metrics.example.com'
STATSD_PORT = '8125'
}
stages {
stage('Build') {
steps {
script {
def startTime = System.currentTimeMillis()
try {
// Record build attempt
sendMetric('build.attempt', 1)
// Perform build
sh 'mvn clean package'
// Record build success
sendMetric('build.success', 1)
// Record build duration
def duration = System.currentTimeMillis() - startTime
sendMetric('build.duration', duration)
} catch (Exception e) {
// Record build failure
sendMetric('build.failure', 1)
throw e
}
}
}
}
stage('Test') {
steps {
script {
def testMetrics = collectTestMetrics()
recordTestResults(testMetrics)
}
}
}
}
}
// Helper function to send metrics
def sendMetric(String name, def value) {
def metricName = "${METRIC_PREFIX}.${name}"
// Send metric to StatsD
sh """
echo "${metricName}:${value}|g" | nc -u -w1 ${STATSD_HOST} ${STATSD_PORT}
"""
// Log metric for debugging
echo "Sent metric: ${metricName} = ${value}"
}
// Function to collect test metrics
def collectTestMetrics() {
def metrics = [:]
// Parse test results
def testResults = junit testResults: '**/target/surefire-reports/*.xml',
allowEmptyResults: true
metrics.totalTests = testResults.totalCount
metrics.passedTests = testResults.passCount
metrics.failedTests = testResults.failCount
metrics.skippedTests = testResults.skipCount
// Calculate pass rate
metrics.passRate = (metrics.totalTests > 0) ?
(metrics.passedTests / metrics.totalTests * 100).round(2) : 0
return metrics
}
// Function to record test results
def recordTestResults(Map metrics) {
// Send test metrics
metrics.each { metric, value ->
sendMetric("tests.${metric}", value)
}
// Generate test report
generateTestReport(metrics)
}
// Function to generate test report
def generateTestReport(Map metrics) {
def report = """
Test Results Summary
-------------------
Total Tests: ${metrics.totalTests}
Passed: ${metrics.passedTests}
Failed: ${metrics.failedTests}
Skipped: ${metrics.skippedTests}
Pass Rate: ${metrics.passRate}%
""".stripIndent()
// Write report to file
writeFile file: 'test-report.txt', text: report
// Archive report
archiveArtifacts artifacts: 'test-report.txt'
}
Hack #8: Implementing Advanced Parallel Execution
Parallel execution in Jenkins is like orchestrating a well-coordinated team where different members can work simultaneously on different tasks. When implemented properly, it can significantly reduce your pipeline execution time. Let’s explore how to implement advanced parallel execution strategies:
pipeline {
agent any
environment {
// Configuration for parallel execution
MAX_PARALLEL_BRANCHES = 4
TEST_SEGMENTS = 8
DEPLOYMENT_ENVIRONMENTS = ['dev', 'staging', 'qa']
}
stages {
stage('Parallel Build and Test') {
parallel {
stage('Build Application') {
steps {
script {
def buildStart = System.currentTimeMillis()
// Execute build process
sh 'mvn clean package -DskipTests'
// Record build timing
def buildDuration = System.currentTimeMillis() - buildStart
echo "Build completed in ${buildDuration}ms"
}
}
}
stage('Run Tests') {
steps {
script {
// Divide tests into segments for parallel execution
def testClasses = findTestClasses()
def testSegments = distributeTests(testClasses, TEST_SEGMENTS)
// Execute test segments in parallel
def parallelTests = [:]
testSegments.eachWithIndex { segment, index ->
parallelTests["Test Segment ${index + 1}"] = {
runTestSegment(segment, index)
}
}
// Execute parallel test segments
parallel parallelTests
}
}
}
stage('Static Analysis') {
steps {
parallel (
"Code Style": {
sh 'checkstyle:checkstyle'
},
"Code Coverage": {
sh 'jacoco:report'
},
"Security Scan": {
sh 'dependency-check:check'
}
)
}
}
}
}
stage('Parallel Deployments') {
steps {
script {
def deployments = [:]
// Create parallel deployment jobs
DEPLOYMENT_ENVIRONMENTS.each { env ->
deployments["Deploy to ${env}"] = {
deployToEnvironment(env)
}
}
// Execute deployments with controlled parallelism
parallel deployments
}
}
}
}
}
// Helper function to find test classes
def findTestClasses() {
def testClasses = []
// Find all test classes in the project
def testFiles = findFiles(glob: '**/src/test/java/**/*Test.java')
testFiles.each { file ->
// Convert file path to class name
def className = file.path
.replaceAll('src/test/java/', '')
.replaceAll('/', '.')
.replaceAll('.java', '')
testClasses << className
}
return testClasses
}
// Helper function to distribute tests across segments
def distributeTests(List testClasses, int segments) {
def distribution = []
def classesPerSegment = (testClasses.size() / segments).ceil()
// Create segments
testClasses.collate(classesPerSegment).each { classes ->
distribution << classes
}
return distribution
}
// Helper function to run test segment
def runTestSegment(List testClasses, int segmentIndex) {
// Create test inclusion pattern
def testPattern = testClasses.join(',')
// Execute tests for this segment
try {
sh """
mvn test \
-Dtest=${testPattern} \
-DforkCount=2 \
-DreuseForks=true \
-Dparallel=classes \
-DthreadCount=4
"""
} catch (Exception e) {
echo "Test segment ${segmentIndex + 1} failed: ${e.message}"
throw e
}
}
// Helper function for environment deployment
def deployToEnvironment(String environment) {
echo "Starting deployment to ${environment}"
try {
// Perform environment-specific deployment steps
stage("Deploy ${environment}") {
// Set environment-specific variables
def envConfig = loadEnvironmentConfig(environment)
// Execute deployment
withCredentials([
string(credentialsId: "${environment}-credentials",
variable: 'DEPLOY_TOKEN')
]) {
sh """
./deploy.sh \
--env ${environment} \
--config ${envConfig} \
--token ${DEPLOY_TOKEN}
"""
}
}
// Verify deployment
stage("Verify ${environment}") {
verifyDeployment(environment)
}
echo "Deployment to ${environment} completed successfully"
} catch (Exception e) {
echo "Deployment to ${environment} failed: ${e.message}"
throw e
}
}
// Helper function to verify deployment
def verifyDeployment(String environment) {
def maxRetries = 5
def retryDelay = 10
for (int i = 0; i < maxRetries; i++) {
try {
sh """
curl -sf https://${environment}.example.com/health
"""
echo "Deployment verification successful"
return
} catch (Exception e) {
if (i == maxRetries - 1) {
error "Deployment verification failed after ${maxRetries} attempts"
}
sleep retryDelay
}
}
}
This implementation of parallel execution demonstrates several important concepts:
Intelligent Test Distribution: The code divides tests into balanced segments for parallel execution, ensuring optimal resource utilization.
Controlled Parallelism: It manages the number of parallel executions to prevent overwhelming the system while maintaining efficiency.
Environment-specific Deployments: The code handles parallel deployments to different environments while maintaining proper isolation and verification.
Error Handling: Comprehensive error handling ensures failures in one parallel branch don’t affect others unnecessarily.
Resource Management: The implementation considers system resources when determining the degree of parallelism.
Master this concept through Master Ansible: Tips, Tricks, and Hacks for Secure and Efficient Automation
Hack #9: Advanced Pipeline Recovery and Self-Healing
The final hack focuses on making Jenkins pipelines more resilient and self-healing. Think of this as giving your pipeline the ability to recover from common problems automatically, much like how a self-driving car can navigate around obstacles. This approach reduces manual intervention and improves pipeline reliability.
pipeline {
agent any
environment {
MAX_RETRIES = 3
RETRY_DELAY = 60 // seconds
HEALTH_CHECK_INTERVAL = 30 // seconds
RECOVERY_LOG = 'recovery-actions.log'
}
options {
// Enable pipeline recovery
timeout(time: 2, unit: 'HOURS')
retry(3)
}
stages {
stage('Pipeline Health Check') {
steps {
script {
// Initialize recovery log
writeFile file: RECOVERY_LOG, text: "Recovery Log - ${new Date()}\n"
// Perform system health check
performHealthCheck()
}
}
}
stage('Build with Recovery') {
steps {
script {
def buildAttempt = 1
def buildSuccess = false
while (!buildSuccess && buildAttempt <= MAX_RETRIES) {
try {
// Attempt the build
sh 'mvn clean package'
buildSuccess = true
logRecoveryAction("Build succeeded on attempt ${buildAttempt}")
} catch (Exception e) {
logRecoveryAction("Build failed on attempt ${buildAttempt}: ${e.message}")
if (buildAttempt < MAX_RETRIES) {
// Implement recovery actions
performRecoveryActions(buildAttempt, e)
buildAttempt++
// Wait before retry
sleep(RETRY_DELAY)
} else {
error "Build failed after ${MAX_RETRIES} attempts"
}
}
}
}
}
}
stage('Deploy with Monitoring') {
steps {
script {
def deploymentMonitor = [
startTime: System.currentTimeMillis(),
healthChecks: [:],
issues: []
]
try {
// Deploy with continuous monitoring
parallel(
"Deployment": {
performDeployment()
},
"Health Monitoring": {
monitorDeploymentHealth(deploymentMonitor)
}
)
} catch (Exception e) {
handleDeploymentFailure(e, deploymentMonitor)
}
}
}
}
}
post {
always {
// Archive recovery logs
archiveArtifacts artifacts: RECOVERY_LOG
// Clean up recovery resources
cleanupRecoveryResources()
}
}
}
// Health check implementation
def performHealthCheck() {
def healthStatus = [:]
// Check Jenkins system health
healthStatus.systemMemory = sh(
script: 'free -m | grep Mem | awk \'{print $4}\'',
returnStdout: true
).trim().toInteger()
healthStatus.diskSpace = sh(
script: 'df -h / | tail -1 | awk \'{print $5}\' | sed \'s/%//\'',
returnStdout: true
).trim().toInteger()
// Verify build tools
healthStatus.mavenStatus = sh(
script: 'mvn --version >/dev/null 2>&1',
returnStatus: true
) == 0
// Check for critical issues
def criticalIssues = healthStatus.findAll { k, v ->
(k == 'systemMemory' && v < 1024) || // Less than 1GB free memory
(k == 'diskSpace' && v > 90) || // More than 90% disk usage
(k == 'mavenStatus' && !v) // Maven not working
}
if (criticalIssues) {
handleCriticalHealthIssues(criticalIssues)
}
}
// Recovery actions implementation
def performRecoveryActions(int attempt, Exception error) {
logRecoveryAction("Initiating recovery actions for attempt ${attempt}")
// Analyze error and determine recovery strategy
def recoveryStrategy = determineRecoveryStrategy(error)
// Execute recovery strategy
switch (recoveryStrategy) {
case 'CLEAN_WORKSPACE':
cleanWorkspace()
break
case 'RESET_MAVEN':
resetMavenRepository()
break
case 'CLEAR_CACHE':
clearBuildCache()
break
default:
logRecoveryAction("No specific recovery strategy for: ${error.message}")
}
}
// Deployment monitoring implementation
def monitorDeploymentHealth(Map monitor) {
while (true) {
// Collect health metrics
def health = checkApplicationHealth()
monitor.healthChecks[System.currentTimeMillis()] = health
// Analyze health trends
analyzeHealthTrends(monitor)
// Take corrective action if needed
if (health.status == 'DEGRADED') {
handleDegradedHealth(health, monitor)
}
sleep(HEALTH_CHECK_INTERVAL)
}
}
// Health trend analysis
def analyzeHealthTrends(Map monitor) {
def recentChecks = monitor.healthChecks.takeRight(5)
// Analyze response time trend
def responseTimes = recentChecks.collect { it.responseTime }
def avgResponseTime = responseTimes.sum() / responseTimes.size()
// Analyze error rate trend
def errorRates = recentChecks.collect { it.errorRate }
def avgErrorRate = errorRates.sum() / errorRates.size()
// Check for concerning trends
if (avgResponseTime > 1000 || avgErrorRate > 0.05) {
logRecoveryAction("""
Concerning health trends detected:
- Average Response Time: ${avgResponseTime}ms
- Average Error Rate: ${avgErrorRate * 100}%
""".stripIndent())
// Implement corrective actions based on trends
implementCorrectiveActions(avgResponseTime, avgErrorRate)
}
}
// Logging utility
def logRecoveryAction(String message) {
def timestamp = new Date().format("yyyy-MM-dd HH:mm:ss")
def logMessage = "${timestamp} - ${message}\n"
// Append to recovery log
writeFile file: RECOVERY_LOG, text: logMessage, append: true
// Also echo to console
echo message
}
// Resource cleanup
def cleanupRecoveryResources() {
// Clean up temporary files
sh "find . -name '*.tmp' -type f -delete"
// Reset environment if needed
if (fileExists('.recovery-lock')) {
sh "rm .recovery-lock"
}
}
This implementation of pipeline recovery and self-healing demonstrates several important concepts:
Proactive Health Monitoring: The pipeline continuously monitors system and application health, allowing it to detect issues before they cause failures.
Intelligent Recovery: The code implements different recovery strategies based on the type of failure encountered, much like how a doctor might prescribe different treatments for different ailments.
Trend Analysis: The implementation analyzes health trends over time, allowing it to identify and address degrading performance before it becomes critical.
Automated Corrective Actions: When issues are detected, the pipeline can automatically implement corrective actions, reducing the need for manual intervention.
Comprehensive Logging: All recovery actions are logged, providing a clear audit trail of what actions were taken and why.
Let’s look at a practical example of how this might work in a real scenario. Imagine your build starts failing due to insufficient memory. The pipeline would:
- Detect the memory issue through health monitoring
- Log the issue in the recovery log
- Attempt to free up memory by cleaning the workspace
- Retry the build with the cleaned workspace
- Continue monitoring to ensure the issue doesn’t recur
This approach transforms Jenkins from a simple automation tool into a self-healing system that can maintain itself and recover from common issues automatically.
Delve into specifics at Building Efficient Pipelines with Jinja2 and AWS S3: A Complete Guide
Conclusion
These nine Jenkins hacks represent a comprehensive approach to building more robust, efficient, and maintainable CI/CD pipelines. From basic configuration improvements to advanced self-healing capabilities, each hack builds upon the others to create a more powerful and reliable Jenkins implementation.
Remember that the key to successful implementation lies in understanding not just how to implement these hacks, but why they’re beneficial and how they work together. Start with the basics and gradually incorporate more advanced features as your team becomes comfortable with each improvement.
External Resources:
Similar Articles
Related Content
More from development
Learn essential Jenkins Versions strategies to optimize your CI/CD pipelines. This guide covers …
Explore the fascinating history of the ls command from its Unix origins to modern Linux …
You Might Also Like
Master Jenkins UserRemoteConfig for dynamic Git repository management. Includes Groovy examples, …
Contents
- Introduction to Jenkins Hacks
- Hack #1: Mastering Jenkins Pipeline Configuration
- Hack #2: Pre-Installing Essential Jenkins Plugins
- Hack #3: Custom Port Configuration for Enhanced Security
- Hack #4: Implementing Shared Libraries
- Hack #5: Advanced Environment Variable Management
- Hack #6: Advanced Security Configuration
- Hack #7: Advanced Pipeline Monitoring and Metrics
- Hack #8: Implementing Advanced Parallel Execution
- Hack #9: Advanced Pipeline Recovery and Self-Healing
- Conclusion