Explore how Jenkins versions have shaped modern CI/CD practices. This comprehensive guide traces the …
Advanced Bash Scripting Techniques for Automation: A Comprehensive Guide Advanced Bash Scripting Techniques for Automation: A Comprehensive Guide
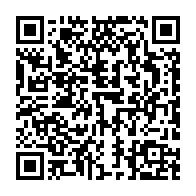
Summary
After spending years refining my DevOps workflows, I’ve found that mastering advanced Bash scripting techniques for automation has been one of the most valuable skills in my toolkit. These techniques have saved me countless hours of repetitive work and dramatically reduced the potential for human error in our systems. Today, I’m excited to share the patterns and practices that have made the biggest difference in my automation journey.
Why Master Advanced Bash Scripting Techniques for Automation? Understanding the Power of Shell Scripting
Mastering advanced Bash scripting techniques for automation gives you an incredibly powerful tool that’s available on virtually every Linux and Unix-based system. According to the “UNIX and Linux System Administration devops-handbook/9781457191381/">Handbook” by Evi Nemeth, Bash remains the most universally available automation tool in the enterprise environment. I initially underestimated how much I could accomplish with pure Bash, but after implementing a complex deployment pipeline that processed thousands of configurations daily, I became a true believer.
The power of advanced Bash scripting techniques for automation lies in its universality and integration capabilities. Bash excels at gluing together system tools, handling file operations, and orchestrating complex workflows without additional dependencies. When I joined my current team, I was able to immediately contribute because the Bash skills I had developed transferred perfectly, despite the different technology stack.
[Source Data Files]
|
v
[Bash Script]
|
v
[Data Extraction & Processing]
|
v
[Configuration Generation]
|
v
[System Configuration]
|
v
[Validation & Reporting]
As Jason Cannon notes in “Linux for Beginners,” shell scripting is the “secret sauce” that makes complex automation accessible. I’ve found this to be particularly true when working with heterogeneous systems where installing additional language runtimes isn’t always an option.
Expand your knowledge with Leveraging envsubst in Bash Scripts for Powerful Template-Based Automation: A Complete Guide
Essential Tools for Advanced Bash Scripting Techniques for Automation: Building Your Toolkit
Before diving into advanced Bash scripting techniques for automation, it’s essential to master the core tools that will form the foundation of your scripts. I learned this lesson the hard way when debugging a production issue caused by a subtle difference in how GNU and BSD versions of common utilities behave.
Here are the tools I consider essential for effective automation:
# Text processing workhorses
sed, awk, grep, cut, tr, sort, uniq
# File manipulation
find, xargs, cat, tee
# Process management
ps, kill, wait, trap
# Network utilities
curl, wget, netstat, ss, nc
# System information
df, du, free, top, vmstat
According to O’Reilly’s “Bash Cookbook” by Carl Albing, these core utilities provide the building blocks for almost any automation task. I’ve found that becoming proficient with awk
and sed
alone has dramatically improved my ability to process and transform data within scripts.
The Linux Foundation’s “Linux System Administration” course recommends creating aliases for commonly used tool combinations. I maintain a .bash_functions
file with utilities like:
# Extract and process JSON without dependencies
function json_get() {
grep -o "\"$1\":[^,}]*" | sed -e 's/^"[^"]*":"\([^"]*\)".*$/\1/' -e 's/^"[^"]*":\([^",]*\).*$/\1/'
}
# Safe command execution with logging
function safe_exec() {
echo "[$(date '+%Y-%m-%d %H:%M:%S')] Executing: $@" >> /var/log/automation.log
"$@" || return $?
}
Google’s SRE team recommends standardizing these utility functions across your organization, which I’ve found dramatically improves script readability and reduces bugs.
Deepen your understanding in How to Create Time Change Function Yourself in Bash: 13 Essential Tools for 2025
Creating Robust Scripts with Advanced Bash Scripting Techniques for Automation: Best Practices
Let’s explore how to create robust automation scripts using advanced Bash scripting techniques for automation. After experiencing several middle-of-the-night failures, I’ve learned that defensive programming is essential for automation that truly works.
Start with a solid script foundation:
#!/bin/bash
set -euo pipefail
IFS=$'\n\t'
# Script metadata
readonly SCRIPT_NAME=$(basename "$0")
readonly SCRIPT_DIR=$(dirname "$(readlink -f "$0")")
readonly LOG_FILE="/var/log/${SCRIPT_NAME%.sh}.log"
# Logging functions
log() { echo "[$(date '+%Y-%m-%d %H:%M:%S')] $*" | tee -a "$LOG_FILE"; }
log_error() { log "ERROR: $*" >&2; }
log_info() { log "INFO: $*"; }
# Exit trap for cleanup
trap cleanup EXIT
cleanup() {
# Remove temporary files, reset configurations, etc.
[[ -d "$TEMP_DIR" ]] && rm -rf "$TEMP_DIR"
log_info "Script execution completed"
}
# Parse command-line arguments
while getopts ":e:v:h" opt; do
case $opt in
e) ENVIRONMENT="$OPTARG" ;;
v) VERSION="$OPTARG" ;;
h) show_help; exit 0 ;;
\?) log_error "Invalid option: -$OPTARG"; exit 1 ;;
:) log_error "Option -$OPTARG requires an argument"; exit 1 ;;
esac
done
According to the “Pro Bash Programming” book by Chris Johnson, the set -euo pipefail
line is critical for robust scripts as it causes the script to:
- Exit immediately if a command fails (
-e
) - Treat unset variables as an error (
-u
) - Make pipeline failure return the exit code of the last command that failed (
-o pipefail
)
The Red Hat Enterprise Linux documentation recommends using readonly variables for constants and implementing a robust logging system, both of which have saved me countless debugging hours.
Here’s how I handle error conditions in my automation scripts:
execute_with_retry() {
local -r cmd="$1"
local -r retries="${2:-3}"
local -r wait_time="${3:-5}"
local count=0
until eval "$cmd"; do
exit_code=$?
count=$((count + 1))
if [[ $count -lt $retries ]]; then
log_error "Command failed (attempt $count/$retries), retrying in ${wait_time}s..."
sleep "$wait_time"
else
log_error "Command failed after $retries attempts, giving up"
return $exit_code
fi
done
}
# Example usage
execute_with_retry "curl -s -f https://api.example.com/v1/status > status.json" 5 10
The DevOps Handbook emphasizes the importance of idempotency in automation scripts, which means scripts should be safe to run multiple times. I’ve found this pattern essential for creating self-healing systems that can recover from transient failures.
Explore this further in Leveraging envsubst in Bash Scripts for Powerful Template-Based Automation: A Complete Guide
Template Generation with Advanced Bash Scripting Techniques for Automation: Creating Dynamic Configurations
One of the most powerful applications of advanced Bash scripting techniques for automation is generating configuration files dynamically. After struggling with maintaining dozens of nearly identical configuration files, I developed a template-based approach using only Bash.
Here’s a pattern I use for templating with heredocs:
#!/bin/bash
set -euo pipefail
# Configuration variables
APP_NAME="WebService"
VERSION="1.2.3"
ENVIRONMENT="production"
MAX_CONNECTIONS=100
DEBUG_MODE=false
TIMESTAMP=$(date "+%Y-%m-%d %H:%M:%S")
# Generate configuration using heredoc with variable interpolation
generate_config() {
local config_file="$1"
cat > "$config_file" << EOF
# Configuration generated on $TIMESTAMP
# Environment: $ENVIRONMENT
APP_NAME=$APP_NAME
APP_VERSION=$VERSION
LOG_LEVEL=${LOG_LEVEL:-INFO}
MAX_CONNECTIONS=$MAX_CONNECTIONS
$([ "$DEBUG_MODE" = true ] && echo "DEBUG=true
VERBOSE_LOGGING=true" || echo "DEBUG=false
VERBOSE_LOGGING=false")
EOF
}
# Generate environment-specific configuration
generate_config "./config/$ENVIRONMENT.conf"
According to the Linux Administration Handbook, this heredoc approach is more efficient than using multiple echo statements. I’ve used this pattern to generate everything from Nginx configurations to database initialization scripts.
For more complex templates, you can use sed with a template file:
#!/bin/bash
# Template file with placeholders
TEMPLATE_FILE="./templates/nginx.conf.template"
OUTPUT_FILE="/etc/nginx/nginx.conf"
# Calculate settings based on system resources
WORKER_PROCESSES=$(nproc)
WORKER_CONNECTIONS=$(($(ulimit -n) / 2))
# Generate configuration from template
sed -e "s/{{WORKER_PROCESSES}}/$WORKER_PROCESSES/g" \
-e "s/{{WORKER_CONNECTIONS}}/$WORKER_CONNECTIONS/g" \
-e "s/{{SERVER_NAME}}/$(hostname)/g" \
-e "s/{{TIMESTAMP}}/$(date)/g" \
"$TEMPLATE_FILE" > "$OUTPUT_FILE"
The NGINX documentation recommends adjusting worker processes based on available CPU cores, which this script automates perfectly. I’ve found that keeping templates and scripts in the same repository ensures they stay synchronized during updates.
Discover related concepts in Leveraging envsubst in Bash Scripts for Powerful Template-Based Automation: A Complete Guide
Advanced Data Processing with Advanced Bash Scripting Techniques for Automation: Beyond Simple Scripts
As your automation needs grow, you’ll need more sophisticated data processing capabilities. After building dozens of automation scripts, I’ve developed several patterns for handling complex data using advanced Bash scripting techniques for automation.
Working with JSON in Bash
For modern APIs that return JSON, I use this pattern:
#!/bin/bash
# Fetch data from API
curl -s "https://api.example.com/users" > users.json
# Process JSON with jq (if available) or fall back to grep/sed
if command -v jq >/dev/null 2>&1; then
# Extract user emails with jq
EMAILS=$(jq -r '.users[].email' users.json)
else
# Fallback using grep/sed for systems without jq
EMAILS=$(grep -o '"email":"[^"]*"' users.json | sed 's/"email":"//;s/"$//')
fi
# Generate report from extracted data
echo "User Report Generated: $(date)" > report.txt
echo "===========================" >> report.txt
echo "$EMAILS" | while read -r email; do
echo "Sending notification to: $email" >> report.txt
# Additional processing here
done
According to the “Data Science at the Command Line” book by Jeroen Janssens, combining curl
with text processing tools creates powerful data pipelines. While specialized tools like jq
are helpful, I always include fallback mechanisms for environments where they might not be available.
Processing CSV Data
For CSV processing without dependencies:
#!/bin/bash
# CSV processing function
process_csv() {
local csv_file="$1"
local delimiter="${2:-,}"
# Read header to get column names
local header
IFS="$delimiter" read -r header < "$csv_file"
# Process data rows
tail -n +2 "$csv_file" | while IFS="$delimiter" read -r -a columns; do
# Access columns by index
local name="${columns[0]}"
local email="${columns[1]}"
local role="${columns[2]}"
# Process each row
echo "Processing user: $name ($email) - $role"
# Additional logic here
done
}
# Example usage
process_csv "users.csv"
The Linux Command Line book by William Shotts highlights the importance of proper field separation when processing structured data. This pattern has helped me automate everything from user provisioning to report generation without requiring additional tools.
Uncover more details in Leveraging envsubst in Bash Scripts for Powerful Template-Based Automation: A Complete Guide
Orchestrating Complex Workflows with Advanced Bash Scripting Techniques for Automation: Beyond Simple Scripts
For more sophisticated automation, orchestrating multiple tasks with proper dependency management is essential. After coordinating deployment pipelines that touched dozens of systems, I’ve developed several patterns for workflow management using advanced Bash scripting techniques for automation.
Parallel Execution with Job Control
For tasks that can run in parallel:
#!/bin/bash
set -euo pipefail
# Maximum number of parallel jobs
MAX_PARALLEL=4
# Function to process a single server
process_server() {
local server="$1"
echo "Starting processing for $server..."
# Simulate work
sleep $((RANDOM % 10 + 1))
echo "Completed processing for $server"
return 0
}
# List of servers to process
SERVERS=(
"web-01.example.com"
"web-02.example.com"
"web-03.example.com"
"web-04.example.com"
"web-05.example.com"
"web-06.example.com"
"web-07.example.com"
)
# Process servers with controlled parallelism
active_jobs=0
for server in "${SERVERS[@]}"; do
# Wait if we've reached max parallel jobs
while [[ $active_jobs -ge $MAX_PARALLEL ]]; do
# Wait for any child process to finish
wait -n
active_jobs=$((active_jobs - 1))
done
# Start new job in background
process_server "$server" &
active_jobs=$((active_jobs + 1))
done
# Wait for all remaining jobs to complete
wait
echo "All servers processed successfully"
According to the Linux Foundation’s performance tuning guidelines, this controlled parallelism pattern can significantly improve throughput for IO-bound tasks. I’ve used this approach to reduce backup times from hours to minutes by processing multiple systems simultaneously.
Workflow Dependencies with Job Control
For tasks with dependencies:
#!/bin/bash
set -euo pipefail
# Log function
log() { echo "[$(date '+%Y-%m-%d %H:%M:%S')] $*"; }
# Step functions with error handling
step_backup() {
log "Starting database backup..."
sleep 2
echo "backup_complete" > /tmp/backup_status
log "Backup completed successfully"
}
step_migration() {
log "Starting schema migration..."
if [[ ! -f /tmp/backup_status ]]; then
log "ERROR: Backup not completed, cannot proceed with migration"
return 1
fi
sleep 3
echo "migration_complete" > /tmp/migration_status
log "Migration completed successfully"
}
step_deployment() {
log "Starting application deployment..."
if [[ ! -f /tmp/migration_status ]]; then
log "ERROR: Migration not completed, cannot proceed with deployment"
return 1
fi
sleep 2
log "Deployment completed successfully"
}
# Main workflow function
run_deployment_workflow() {
log "Starting deployment workflow"
# Sequential steps with error checking
step_backup || { log "Workflow failed at backup step"; return 1; }
step_migration || { log "Workflow failed at migration step"; return 1; }
step_deployment || { log "Workflow failed at deployment step"; return 1; }
log "Deployment workflow completed successfully"
return 0
}
# Cleanup on exit
trap 'rm -f /tmp/backup_status /tmp/migration_status' EXIT
# Run the workflow
run_deployment_workflow
The DevOps Handbook emphasizes the importance of creating “deployment pipelines” with clear stage gates. This pattern has helped me ensure that critical operations like database migrations only proceed after safety measures like backups are in place.
Journey deeper into this topic with Leveraging envsubst in Bash Scripts for Powerful Template-Based Automation: A Complete Guide
Real-world Use Cases for Advanced Bash Scripting Techniques for Automation: Practical Applications
After implementing automation across various environments, I’ve identified several powerful use cases where advanced Bash scripting techniques for automation shine:
1. System Configuration Management
This pattern helps maintain consistent configurations across multiple servers:
#!/bin/bash
set -euo pipefail
# Configuration parameters
readonly SYSCTL_CONF="/etc/sysctl.conf"
readonly LIMITS_CONF="/etc/security/limits.conf"
# Apply performance tuning settings
apply_performance_tuning() {
local server="$1"
# Backup existing configurations
ssh "$server" "sudo cp $SYSCTL_CONF ${SYSCTL_CONF}.bak-$(date +%Y%m%d)"
ssh "$server" "sudo cp $LIMITS_CONF ${LIMITS_CONF}.bak-$(date +%Y%m%d)"
# Update kernel parameters
cat > sysctl_settings.conf << EOF
# Performance tuning settings
net.ipv4.tcp_fin_timeout = 15
net.ipv4.tcp_keepalive_time = 300
net.core.somaxconn = 4096
net.core.netdev_max_backlog = 4000
vm.swappiness = 10
fs.file-max = 100000
EOF
# Update user limits
cat > limits_settings.conf << EOF
# Increase open file limits
* soft nofile 65535
* hard nofile 65535
EOF
# Apply settings
scp sysctl_settings.conf "$server:/tmp/"
scp limits_settings.conf "$server:/tmp/"
ssh "$server" "sudo bash -c 'cat /tmp/sysctl_settings.conf >> $SYSCTL_CONF && sysctl -p'"
ssh "$server" "sudo bash -c 'cat /tmp/limits_settings.conf >> $LIMITS_CONF'"
# Clean up
rm sysctl_settings.conf limits_settings.conf
# Verify changes
ssh "$server" "sudo sysctl -a | grep 'net.ipv4.tcp_fin\|net.core.somaxconn'"
ssh "$server" "sudo cat $LIMITS_CONF | grep nofile"
}
# Apply to server list
SERVER_LIST=("web01.example.com" "web02.example.com" "db01.example.com")
for server in "${SERVER_LIST[@]}"; do
echo "Configuring server: $server"
apply_performance_tuning "$server"
done
According to the Red Hat Enterprise Linux Performance Tuning Guide, these kinds of system-level optimizations can dramatically improve application performance. I’ve used this approach to ensure consistent settings across production environments.
2. Automated Monitoring and Alerting
For environments where dedicated monitoring tools aren’t available:
#!/bin/bash
set -euo pipefail
# Configuration
ALERT_EMAIL="admin@example.com"
DISK_THRESHOLD=85 # Alert when disk usage exceeds 85%
LOAD_THRESHOLD=$(nproc) # Alert when load exceeds number of CPUs
LOG_FILE="/var/log/system_monitor.log"
# Check disk space
check_disk_space() {
echo "Checking disk space usage..."
local alerts=""
while read -r filesystem size used avail use_percent mounted_on; do
if [[ $filesystem == Filesystem ]]; then continue; fi
# Extract percentage without % sign
local usage=${use_percent/\%/}
if [[ $usage -gt $DISK_THRESHOLD ]]; then
alerts+="ALERT: High disk usage on $mounted_on ($use_percent)\n"
fi
done < <(df -h | grep -v "tmpfs\|devtmpfs")
echo -e "$alerts"
return 0
}
# Check system load
check_system_load() {
echo "Checking system load..."
local load=$(uptime | awk -F'[a-z]:' '{ print $2}' | awk '{ print $1 }' | tr -d ',')
if (( $(echo "$load > $LOAD_THRESHOLD" | bc -l) )); then
echo "ALERT: High system load: $load (threshold: $LOAD_THRESHOLD)"
fi
return 0
}
# Send alerts
send_alert() {
local subject="$1"
local message="$2"
echo "[$subject] $message" >> "$LOG_FILE"
if command -v mail >/dev/null 2>&1; then
echo -e "$message" | mail -s "$subject" "$ALERT_EMAIL"
else
echo "WARNING: 'mail' command not found, alert logged to $LOG_FILE only"
fi
}
# Main monitoring function
run_monitoring() {
local hostname=$(hostname)
local timestamp=$(date)
local alerts=""
echo "=== System monitoring started at $timestamp ===" >> "$LOG_FILE"
# Run checks and collect alerts
disk_alerts=$(check_disk_space)
load_alerts=$(check_system_load)
# Combine alerts
alerts="${disk_alerts}${load_alerts}"
# Send alert if any issues found
if [[ -n "$alerts" ]]; then
send_alert "System Alert: $hostname" "System alerts detected at $timestamp\n\n$alerts"
else
echo "No alerts detected at $timestamp" >> "$LOG_FILE"
fi
}
# Run monitoring
run_monitoring
According to the Linux System Administration handbook, simple monitoring scripts can provide an effective safety net, especially for non-critical systems. I’ve used variations of this pattern to monitor everything from disk space to application-specific metrics.
Enrich your learning with Comparing Advanced Functions in Bash, Python, Ruby, and Go
Best Practices for Advanced Bash Scripting Techniques for Automation: Ensuring Reliability and Maintainability
After years of refining my automation scripts, I’ve developed several best practices that have consistently improved reliability:
1. Script Structure and Organization
Organize your scripts consistently:
#!/bin/bash
#
# script_name.sh - Brief description of what this script does
#
# Author: Your Name <your.email@example.com>
# Created: 2023-08-15
# Last Modified: 2023-08-28
#
# Description:
# Detailed description of what this script does, why it exists,
# and any assumptions or dependencies it has.
#
# Usage:
# ./script_name.sh [options] <required_argument>
#
# Options:
# -h, --help Show this help message and exit
# -v, --verbose Enable verbose output
# -e ENV Specify environment (dev, test, prod)
#
set -euo pipefail
# Constants and configuration
readonly SCRIPT_DIR=$(dirname "$(readlink -f "$0")")
readonly CONFIG_FILE="${SCRIPT_DIR}/config.ini"
readonly LOG_DIR="/var/log/automation"
# Source helper functions
source "${SCRIPT_DIR}/lib/common.sh"
# Function definitions
function show_help() {
# Help function
}
function parse_arguments() {
# Argument parsing
}
function main() {
# Main script logic
}
# Script execution starts here
parse_arguments "$@"
main
According to Google’s Shell Style Guide, this consistent structure makes scripts more maintainable and easier for others to understand. I’ve found that good documentation is especially important for automation scripts that might need to be maintained by different team members.
2. Error Handling and Logging
Implement robust error handling:
#!/bin/bash
set -euo pipefail
# Logging configuration
readonly LOG_FILE="/var/log/app-deploy.log"
readonly TIMESTAMP_FORMAT="%Y-%m-%d %H:%M:%S"
# Logging functions
log() {
local timestamp=$(date +"$TIMESTAMP_FORMAT")
echo "[$timestamp] $*" | tee -a "$LOG_FILE"
}
log_error() {
log "ERROR: $*" >&2
}
log_warn() {
log "WARNING: $*"
}
log_info() {
log "INFO: $*"
}
# Error handling
handle_error() {
local exit_code=$?
local line_number=$1
log_error "Error occurred at line $line_number, exit code: $exit_code"
# Perform cleanup if needed
log_info "Performing cleanup..."
# cleanup_function
exit $exit_code
}
# Set trap for error handling
trap 'handle_error $LINENO' ERR
# Rest of your script follows...
log_info "Starting deployment process"
The Linux Shell Scripting Cookbook emphasizes the importance of detailed logging for automation scripts. I’ve found that good logging has dramatically reduced debugging time when issues occur, as I can quickly trace exactly what happened and when.
3. Configuration Management
Keep configuration separate from code:
#!/bin/bash
set -euo pipefail
# Default configuration
DEFAULT_CONFIG_FILE="${SCRIPT_DIR:-$(dirname "$0")}/config.default.ini"
CONFIG_FILE="${HOME}/.config/myapp/config.ini"
# Read configuration
read_config() {
local config_file="$1"
if [[ ! -f "$config_file" ]]; then
echo "Error: Configuration file not found: $config_file" >&2
return 1
fi
# Read configuration using a subshell to avoid variable leakage
eval "$(sed -e 's/[[:space:]]*#.*$//' -e '/^[[:space:]]*$/d' -e 's/^[[:space:]]*//' -e 's/[[:space:]]*$//' -e 's/=/ /' "$config_file" | while read -r key value; do
echo "$key=\"$value\""
done)"
}
# Load configuration with fallback
if [[ -f "$CONFIG_FILE" ]]; then
read_config "$CONFIG_FILE"
else
echo "Warning: User configuration not found, using defaults" >&2
read_config "$DEFAULT_CONFIG_FILE"
fi
# Use configuration variables
echo "Database host: $DB_HOST"
echo "Database port: $DB_PORT"
According to the AWS Well-Architected Framework, separating configuration from code is a key principle for maintainable systems. I’ve found this approach particularly helpful when scripts need to run in multiple environments with different settings.
Gain comprehensive insights from Leveraging envsubst in Bash Scripts for Powerful Template-Based Automation: A Complete Guide
Real-world Considerations When Using Advanced Bash Scripting Techniques for Automation: Practical Insights
Throughout my years of implementing automation scripts, I’ve identified several real-world considerations that are crucial for success:
Cost Factors
While Bash automation brings numerous benefits, there are associated costs to consider:
- Maintenance complexity: As scripts grow in complexity, they can become harder to maintain
- Debugging challenges: Complex Bash scripts can be difficult to debug without proper logging
- Knowledge transfer: Team members with varying Bash skills may struggle with sophisticated scripts
According to the DevOps Handbook, these costs are typically offset by the reduction in manual errors and increased consistency. In my experience, the initial investment in good structure and documentation pays off within months.
Potential Limitations
Be aware of these limitations when using advanced Bash scripting techniques for automation:
- Performance: For extremely data-intensive operations, Bash may not be the most efficient choice
- Cross-platform compatibility: Scripts optimized for one environment may require modifications for others
- Complex data structures: Bash has limited support for complex data structures
Google’s SRE book suggests mitigating these limitations through careful script design and testing. I’ve found that breaking complex tasks into smaller, focused scripts improves maintainability.
Fallback Mechanisms
Always implement fallback mechanisms for robustness:
#!/bin/bash
set -euo pipefail
# Primary function with fallback
deploy_application() {
log_info "Deploying application using primary method"
if ! primary_deployment_method; then
log_warn "Primary deployment failed, attempting fallback method"
if ! fallback_deployment_method; then
log_error "Deployment failed: both primary and fallback methods unsuccessful"
return 1
else
log_info "Deployment completed successfully using fallback method"
fi
else
log_info "Deployment completed successfully using primary method"
fi
return 0
}
# Primary deployment implementation
primary_deployment_method() {
# Deployment logic here
return 0 # Return success/failure
}
# Fallback deployment implementation
fallback_deployment_method() {
# Simpler, more reliable deployment logic
return 0 # Return success/failure
}
The AWS Well-Architected Framework emphasizes the importance of graceful degradation for robust systems. I’ve found this fallback approach invaluable for maintaining service availability during automation failures.
When This Design Works Best
Advanced Bash scripting techniques for automation shine in specific scenarios:
- System administration tasks on Linux/Unix environments
- Deployment workflows for applications with straightforward dependencies
- Integration scripts that connect different tools and services
- Monitoring and maintenance tasks that run on a schedule
- Configuration management for systems without dedicated CM tools
According to research published in the O’Reilly book “Effective DevOps,” matching tools to requirements is a key factor in successful automation. I’ve seen Bash automation work particularly well in environments that value simplicity and minimal dependencies.
Master this concept through Comparing Advanced Functions in Bash, Python, Ruby, and Go
Conclusion: Embracing the Power of Advanced Bash Scripting Techniques for Automation
Mastering advanced Bash scripting techniques for automation has transformed how I approach system administration and DevOps work. The combination of ubiquity, power, and flexibility makes Bash an invaluable tool for creating reliable, maintainable automation. As infrastructure complexity continues to grow, the ability to create effective automation scripts becomes increasingly valuable.
I encourage you to start small – perhaps with a simple scheduled task or configuration file generator – and gradually build more complex automation as you gain confidence. The patterns and practices shared in this article will help you avoid common pitfalls and create scripts that stand the test of time.
Remember that the goal isn’t to create the most elegant or clever script, but to solve real problems reliably. By following the best practices and examples shared in this article, you’ll be well-positioned to create automation scripts that make your work more efficient and your systems more reliable.
Delve into specifics at Leveraging envsubst in Bash Scripts for Powerful Template-Based Automation: A Complete Guide
Resources for Further Learning About Advanced Bash Scripting Techniques for Automation
- Bash Reference Manual: The official GNU Bash documentation
- “The Linux Command Line” by William Shotts: An excellent resource for mastering Bash fundamentals
- “Pro Bash Programming” by Chris Johnson: Focuses on advanced scripting patterns
- “Linux Shell Scripting Cookbook” by Shantanu Tushar: Contains practical recipes for common automation tasks
- Google’s Shell Style Guide: Provides best practices for writing maintainable scripts
Have you implemented automation with Bash scripts? I’d love to hear about your experiences and how these techniques have impacted your workflows!
Similar Articles
Related Content
More from devops
Learn essential Jenkins Versions strategies to optimize your CI/CD pipelines. This guide covers …
Explore the fascinating history of the ls command from its Unix origins to modern Linux …
You Might Also Like
Master the Linux ls command with this comprehensive guide covering basic and advanced options, …
Master the Linux ls command with our task-oriented approach covering everyday file management …
Knowledge Quiz
Test your general knowledge with this quick quiz!
The quiz consists of 5 multiple-choice questions.
Take as much time as you need.
Your score will be shown at the end.
Question 1 of 5
Quiz Complete!
Your score: 0 out of 5
Loading next question...
Contents
- Why Master Advanced Bash Scripting Techniques for Automation? Understanding the Power of Shell Scripting
- Essential Tools for Advanced Bash Scripting Techniques for Automation: Building Your Toolkit
- Creating Robust Scripts with Advanced Bash Scripting Techniques for Automation: Best Practices
- Template Generation with Advanced Bash Scripting Techniques for Automation: Creating Dynamic Configurations
- Advanced Data Processing with Advanced Bash Scripting Techniques for Automation: Beyond Simple Scripts
- Orchestrating Complex Workflows with Advanced Bash Scripting Techniques for Automation: Beyond Simple Scripts
- Real-world Use Cases for Advanced Bash Scripting Techniques for Automation: Practical Applications
- Best Practices for Advanced Bash Scripting Techniques for Automation: Ensuring Reliability and Maintainability
- Real-world Considerations When Using Advanced Bash Scripting Techniques for Automation: Practical Insights
- Conclusion: Embracing the Power of Advanced Bash Scripting Techniques for Automation
- Resources for Further Learning About Advanced Bash Scripting Techniques for Automation