Complete tutorial on deploying Jenkins to Amazon EKS. Learn what pods are, why deployments matter, …
Jenkins UserRemoteConfig: The Ultimate Guide for Dynamic Git Integration Jenkins UserRemoteConfig: The Ultimate Guide for Dynamic Git Integration
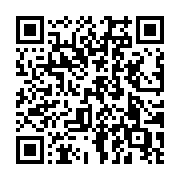
Summary
Master repository management in Jenkins pipelines with UserRemoteConfig, Groovy scripting, and advanced CI/CD workflows
For almost a decade, I’ve automated software delivery pipelines for Fortune 500 companies, and Jenkins UserRemoteConfig remains my go-to tool for dynamic Git repository management. This class—hidden in plain sight within the Jenkins Git Plugin—unlocks unparalleled flexibility for configuring remote repositories, credentials, and branches programmatically. In this guide, I’ll share battle-tested strategies to leverage Jenkins UserRemoteConfig for secure, scalable CI/CD pipelines.
What is Jenkins UserRemoteConfig?
Jenkins UserRemoteConfig is a Java/Groovy class that defines how Jenkins interacts with remote Git repositories. It encapsulates critical parameters like repository URLs, credentials, and branch specifications. Unlike static configurations, UserRemoteConfig enables dynamic adjustments via code, making it indispensable for multi-environment workflows.
Key Components of Jenkins UserRemoteConfig:
Expand your knowledge with Comprehensive Guide to Jenkins Versions: Implementation, Best Practices, and Real-world Examples
url
: The Git repository endpoint (e.g.,https://github.com/org/repo.git
).credentialsId
: Jenkins-stored credentials for authentication.name
: Optional identifier for repository references.
Why Jenkins UserRemoteConfig Matters for Modern DevOps
1. Eliminate Hardcoding with Dynamic Configuration
Hardcoding repository URLs in Jenkinsfiles is a recipe for disaster. Jenkins UserRemoteConfig allows parameterization via:
- Pipeline Inputs: Let users specify repositories at runtime.
- Shared Libraries: Centralize configurations across teams.
Example:
pipeline {
agent any
parameters {
string(name: 'REPO_URL', defaultValue: 'https://github.com/example/repo.git')
}
stages {
stage('Checkout') {
steps {
script {
checkout([
$class: 'GitSCM',
userRemoteConfigs: [
[url: params.REPO_URL, credentialsId: 'github-creds']
],
branches: [[name: 'main']]
])
}
}
}
}
}
Why This Works: Reduces configuration drift and enables reusable pipelines.
2. Multi-Repository Workflows Made Simple
Manage multiple repositories in a single pipeline. For example, pull code from GitHub and push artifacts to GitLab:
checkout([
$class: 'GitSCM',
userRemoteConfigs: [
[url: 'https://github.com/source/repo.git', credentialsId: 'github'],
[url: 'https://gitlab.com/target/repo.git', credentialsId: 'gitlab']
]
])
Use Case: A financial services client uses this pattern to sync code between public and private repositories.
Deepen your understanding in The Evolution of Jenkins Versions: A Journey Through CI/CD History
Advanced Jenkins UserRemoteConfig Techniques
1. Script Console Automation
Bulk-update repository configurations across all Jenkins jobs:
import hudson.plugins.git.UserRemoteConfig
Jenkins.instance.getAllItems(Job.class).each { job ->
def scm = job.scm
if (scm instanceof GitSCM) {
scm.userRemoteConfigs = [
new UserRemoteConfig(
'https://new-repo-url.git',
'credentials-id',
null,
null
)
]
job.save()
}
}
Pro Tip: Use this script during infrastructure migrations.
2. Conditional Branch Selection
Dynamically switch branches based on environment variables:
Explore this further in Comprehensive Guide to Jenkins Versions: Implementation, Best Practices, and Real-world Examples
node {
def BRANCH = env.ENVIRONMENT == 'prod' ? 'main' : 'develop'
checkout([
$class: 'GitSCM',
userRemoteConfigs: [[url: 'https://github.com/repo.git']],
branches: [[name: BRANCH]]
])
}
Jenkins UserRemoteConfig vs. Similar Classes
1. GitSCM
The parent class for Git operations. UserRemoteConfig is a component of GitSCM
that specifically handles remote repository details.
2. CloneOption
A helper class for defining clone behavior (e.g., shallow clones):
checkout([
$class: 'GitSCM',
userRemoteConfigs: [[url: 'https://github.com/repo.git']],
extensions: [
[$class: 'CloneOption', shallow: true, depth: 1]
]
])
3. BranchSpec
Specifies branch patterns to checkout. Pair it with UserRemoteConfig for fine-grained control.
Discover related concepts in Deploy Jenkins on Amazon EKS: Complete Tutorial for Pods and Deployments
Real-World Jenkins UserRemoteConfig Use Cases
Case Study 1: Compliance-Driven Deployments
A healthcare client uses UserRemoteConfig to enforce strict repository access controls, ensuring only approved branches are deployed to production.
Case Study 2: Open-Source Contributions
Automate PR validation by dynamically fetching contributors’ forks:
Uncover more details in Comprehensive Guide to Jenkins Versions: Implementation, Best Practices, and Real-world Examples
checkout([
$class: 'GitSCM',
userRemoteConfigs: [
[url: "https://github.com/${params.CONTRIBUTOR}/repo.git", credentialsId: 'oss-creds']
],
branches: [[name: params.PR_BRANCH]]
])
Best Practices for Jenkins UserRemoteConfig
1. Secure Credential Management
Never hardcode secrets. Use Jenkins’ built-in credential storage:
withCredentials([usernamePassword(credentialsId: 'git-creds', usernameVariable: 'USER', passwordVariable: 'PASS')]) {
sh "git clone https://${USER}:${PASS}@github.com/repo.git"
}
Security Note: Rotate credentials regularly and audit usage.
2. Performance Optimization
Speed up pipelines with shallow clones:
checkout([
$class: 'GitSCM',
userRemoteConfigs: [[url: 'https://github.com/repo.git']],
extensions: [[$class: 'CloneOption', shallow: true, depth: 1]]
])
3. Error Handling
Add retries for flaky connections:
Journey deeper into this topic with Comprehensive Guide to Jenkins Versions: Implementation, Best Practices, and Real-world Examples
retry(3) {
checkout([
$class: 'GitSCM',
userRemoteConfigs: [[url: 'https://github.com/repo.git']]
])
}
Troubleshooting Jenkins UserRemoteConfig
1. Authentication Failures
- Symptom:
Authentication failed
errors. - Fix: Verify
credentialsId
exists in Jenkins → Manage Credentials.
2. Branch Not Found
- Symptom:
Could not find branch
errors. - Fix: Use
refs/heads/
prefix for explicit branch references.
Conclusion: Jenkins UserRemoteConfig as a DevOps Power Tool
After 7 years of automating pipelines, I can confidently say Jenkins UserRemoteConfig is essential for scalable Git integration. By mastering its parameters, Groovy scripting, and security practices, you’ll future-proof your CI/CD workflows.
Next Steps:
- Explore the official Javadoc for advanced methods.
- Experiment with dynamic configurations in a sandbox Jenkins instance.
Similar Articles
Related Content
More from devops
Explore how Jenkins versions have shaped modern CI/CD practices. This comprehensive guide traces the …
Learn essential Jenkins Versions strategies to optimize your CI/CD pipelines. This guide covers …
You Might Also Like
No related topic suggestions found.
Knowledge Quiz
Test your general knowledge with this quick quiz!
The quiz consists of 5 multiple-choice questions.
Take as much time as you need.
Your score will be shown at the end.
Question 1 of 5
Quiz Complete!
Your score: 0 out of 5
Loading next question...
Contents
- What is Jenkins UserRemoteConfig?
- Why Jenkins UserRemoteConfig Matters for Modern DevOps
- Advanced Jenkins UserRemoteConfig Techniques
- Jenkins UserRemoteConfig vs. Similar Classes
- Real-World Jenkins UserRemoteConfig Use Cases
- Best Practices for Jenkins UserRemoteConfig
- Troubleshooting Jenkins UserRemoteConfig
- Conclusion: Jenkins UserRemoteConfig as a DevOps Power Tool