Complete tutorial on deploying Jenkins to Amazon EKS. Learn what pods are, why deployments matter, …
9 Ways Node.js Deployments with CI/CD Pipelines: A Complete Guide 9 Ways Node.js Deployments with CI/CD Pipelines: A Complete Guide
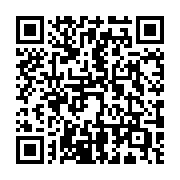
Summary
Introduction
In today’s fast-paced development environment, efficient Node.js Deployments have become a cornerstone of modern software engineering. By integrating continuous integration and continuous delivery (CI/CD) pipelines, developers can automate testing, deployment, and scaling of applications with greater speed and reliability. This comprehensive guide is designed to help you understand the critical aspects of Node.js Deployments with CI/CD Pipelines and to show you nine practical ways to enhance your deployment strategies.
As you begin your journey into CI/CD for Node.js, it’s important to recognize that each deployment method covered here not only improves efficiency but also reinforces quality and security. Whether you’re just starting out or looking to upgrade your existing workflows, this guide will walk you through step-by-step examples, coding snippets, and best practices to ensure you can confidently implement these strategies.
Expand your knowledge with Mastering Database Scaling in Microservices: An Introduction | DevOps
Node.js Deployments: Automated Testing Integration
Effective Node.js Deployments start with automated testing integration. Automated testing is the first line of defense against bugs and regressions. By integrating testing into your CI/CD pipeline, you ensure that only thoroughly vetted code is deployed to production.
Imagine you’re developing an application with unit tests written in Mocha. Each time a change is pushed, your CI tool (such as GitHub Actions or Jenkins) automatically runs these tests. If any test fails, the deployment halts, preventing faulty code from affecting users.
Example: Integrating Mocha Tests in GitHub Actions
Below is an example of a GitHub Actions workflow file (.github/workflows/nodejs-tests.yml
) that runs Mocha tests every time you push code:
name: Node.js CI
on:
push:
branches: [ main ]
pull_request:
branches: [ main ]
jobs:
test:
runs-on: ubuntu-latest
strategy:
matrix:
node-version: [14.x, 16.x]
steps:
- name: Checkout Code
uses: actions/checkout@v2
- name: Setup Node.js
uses: actions/setup-node@v2
with:
node-version: ${{ matrix.node-version }}
- name: Install Dependencies
run: npm install
- name: Run Mocha Tests
run: npm test
Explanation:
- Checkout Code: The workflow begins by checking out your repository.
- Setup Node.js: It then sets up the specified Node.js version.
- Install Dependencies: The command installs project dependencies.
- Run Mocha Tests: Finally, it executes the tests using
npm test
.
If any tests fail, the workflow stops, ensuring only quality code proceeds further. For more details on Mocha, you can visit the Mocha documentation.
Integrating automated testing in your Node.js Deployments helps catch errors early and ensures that each code change is robust and reliable before reaching production.
Deepen your understanding in DevOps Tools in the Industry: A Comprehensive Guide to Mastering the Art of DevOps
Node.js Deployments: Containerization with Docker
Containerization is a powerful strategy in Node.js Deployments that enables you to package your application with all its dependencies into a container. Docker is the industry-standard tool for this purpose. Containers help maintain consistency across different environments, from local development to production servers.
Example: Creating a Dockerfile for a Node.js Application
Below is an example Dockerfile for a basic Node.js application:
# Use the official Node.js 16 image as a parent image
FROM node:16
# Set the working directory
WORKDIR /usr/src/app
# Copy package.json and package-lock.json
COPY package*.json ./
# Install dependencies
RUN npm install --production
# Copy the rest of the application code
COPY . .
# Expose the application port
EXPOSE 3000
# Define the command to run the application
CMD [ "node", "app.js" ]
Explanation:
- FROM node:16: Specifies the base image with Node.js version 16.
- WORKDIR: Sets the working directory in the container.
- COPY & RUN: Copies the dependency files and installs production dependencies.
- COPY: Transfers the remaining code into the container.
- EXPOSE: Opens the port that your application listens on (port 3000 in this example).
- CMD: Defines the command to start your Node.js application.
By containerizing your application, Node.js Deployments become more predictable, reproducible, and scalable. You can integrate Docker builds into your CI/CD pipelines, ensuring that every deployment is packaged and tested consistently. To learn more about Docker, check out the Docker documentation.
Explore this further in Node.js and LangChain: Bridging JavaScript and AI
Node.js Deployments: Environment Variable Management
Managing configuration through environment variables is a key aspect of secure and scalable Node.js Deployments. Environment variables allow you to separate configuration from code, making it easier to manage settings across different deployment environments such as development, staging, and production.
Example: Using dotenv for Environment Variables
A common approach in Node.js is to use the dotenv
package to load environment variables from a .env
file. Here’s a sample configuration:
Install dotenv:
npm install dotenv
Create a
.env
file:PORT=3000 DB_HOST=localhost DB_USER=root DB_PASS=securepassword
Load the .env file in your application (
app.js
):require('dotenv').config(); const express = require('express'); const app = express(); const port = process.env.PORT || 3000; app.get('/', (req, res) => { res.send('Hello, Node.js Deployments with CI/CD Pipelines!'); }); app.listen(port, () => { console.log(`Server is running on port ${port}`); });
Explanation:
- dotenv Configuration: The
require('dotenv').config()
statement loads variables from the.env
file intoprocess.env
. - Using Variables: The application uses these variables to configure the server, ensuring that sensitive information remains outside the source code.
Integrating environment variable management into your Node.js Deployments enhances security and allows for smoother transitions between different environments. For more details, visit the dotenv GitHub repository.
Discover related concepts in Dynamic Configuration Management with envsubst and Jinja2: Simplifying Deployments
Node.js Deployments: Zero Downtime Releases
Zero downtime releases are crucial for maintaining a seamless user experience during updates. Implementing strategies such as blue-green deployments or canary releases ensures that your Node.js Deployments can be updated without interrupting service.
Example: Blue-Green Deployment with PM2
PM2 is a popular process manager for Node.js that supports zero downtime deployments. Here’s how you can set up a blue-green deployment strategy using PM2:
Install PM2:
npm install pm2 -g
Start Your Application:
pm2 start app.js --name "my-app"
Deploy a New Version:
When deploying a new version, you can use PM2’s reload command:
pm2 reload my-app
Explanation:
- PM2 Reload: The reload command allows PM2 to restart your application without downtime by ensuring that a new instance is started before the old one is stopped.
- Blue-Green Strategy: This technique creates two identical environments (blue and green). The live environment (blue) handles traffic while the new version (green) is deployed. Once verified, traffic is switched to the green environment, minimizing downtime.
By adopting zero downtime release strategies, your Node.js Deployments can maintain service availability even during updates. For further details, you can refer to the PM2 documentation.
Uncover more details in DevOps Tools in the Industry: A Comprehensive Guide to Mastering the Art of DevOps
Node.js Deployments: Infrastructure as Code
Infrastructure as Code (IaC) is a practice that allows you to manage and provision infrastructure through code rather than manual processes. This approach is a game-changer for Node.js Deployments as it automates the setup and configuration of servers, databases, and networks, ensuring consistency and scalability.
Example: Using Terraform for Node.js Deployments
Below is a simple example of a Terraform configuration file (main.tf
) that provisions an AWS EC2 instance for a Node.js application:
provider "aws" {
region = "us-east-1"
}
resource "aws_instance" "node_app" {
ami = "ami-0c55b159cbfafe1f0"
instance_type = "t2.micro"
tags = {
Name = "NodeJS-Deployments-Server"
}
provisioner "remote-exec" {
inline = [
"sudo apt-get update",
"curl -sL https://deb.nodesource.com/setup_16.x | sudo -E bash -",
"sudo apt-get install -y nodejs",
"git clone https://github.com/yourusername/your-nodejs-app.git",
"cd your-nodejs-app && npm install && pm2 start app.js"
]
}
}
Explanation:
- Provider Block: Specifies the AWS region and credentials.
- Resource Definition: The
aws_instance
resource creates a new EC2 instance. - Provisioner: Uses a remote-exec provisioner to install Node.js, clone the application repository, install dependencies, and start the app using PM2.
Using IaC tools like Terraform in your Node.js Deployments ensures that your infrastructure is version-controlled, repeatable, and scalable. Learn more about Terraform on their official website.
Journey deeper into this topic with Mastering Node.js Error Handling: Building Resilient Applications
Node.js Deployments: Rolling Updates Strategy
Rolling updates allow you to update your application incrementally, ensuring that a portion of your users always has access to a stable version of your app. This strategy is particularly valuable for Node.js Deployments where high availability is essential.
Example: Implementing Rolling Updates with Kubernetes
Consider a scenario where your Node.js application is deployed on Kubernetes. A rolling update can be performed by updating the deployment configuration:
apiVersion: apps/v1
kind: Deployment
metadata:
name: nodejs-deployment
spec:
replicas: 3
strategy:
type: RollingUpdate
rollingUpdate:
maxUnavailable: 1
maxSurge: 1
selector:
matchLabels:
app: nodejs-app
template:
metadata:
labels:
app: nodejs-app
spec:
containers:
- name: nodejs-container
image: yourdockerhub/nodejs-app:latest
ports:
- containerPort: 3000
Explanation:
- Deployment Configuration: Defines a Kubernetes deployment for your Node.js application.
- Rolling Update Strategy: Specifies the maximum number of pods that can be unavailable or in excess during the update process.
- Container Image Update: Deploys a new image version, ensuring that only a small percentage of pods are updated at once.
This rolling update mechanism in your CI/CD pipeline allows for smooth transitions between application versions, reducing the risk of downtime. For more on Kubernetes rolling updates, see the Kubernetes documentation.
Enrich your learning with DevOps Tools in the Industry: A Comprehensive Guide to Mastering the Art of DevOps
Node.js Deployments: GitOps for Automation
GitOps is a modern approach that uses Git as the single source of truth for your infrastructure and application configurations. By automating deployments through pull requests and code reviews, GitOps practices reinforce the stability and security of your Node.js Deployments.
Example: GitHub Actions Workflow for GitOps
Below is an example GitHub Actions workflow (.github/workflows/gitops-deploy.yml
) that automatically deploys a Node.js application when changes are merged into the main branch:
name: GitOps Node.js Deployment
on:
push:
branches: [ main ]
jobs:
deploy:
runs-on: ubuntu-latest
steps:
- name: Checkout Repository
uses: actions/checkout@v2
- name: Setup Node.js
uses: actions/setup-node@v2
with:
node-version: 16
- name: Install Dependencies
run: npm install
- name: Build Application
run: npm run build
- name: Deploy to Production
env:
SSH_PRIVATE_KEY: ${{ secrets.SSH_PRIVATE_KEY }}
run: |
echo "$SSH_PRIVATE_KEY" > key.pem
chmod 600 key.pem
rsync -avz -e "ssh -i key.pem" ./build/ user@yourserver.com:/var/www/nodejs-app
Explanation:
- Checkout & Setup: The workflow checks out the repository and sets up the Node.js environment.
- Build and Deployment: It builds the application and deploys the build directory to a production server using
rsync
over SSH. - GitOps Approach: By relying on Git events to trigger deployments, this workflow automates the delivery process while ensuring transparency and version control.
This GitOps strategy integrates seamlessly with your CI/CD pipeline, streamlining Node.js Deployments by leveraging familiar tools like GitHub Actions. For further reading, explore the GitOps documentation.
Gain comprehensive insights from Master Ansible: Tips, Tricks, and Hacks for Secure and Efficient Automation
Node.js Deployments: Monitoring and Logging Integration
Robust monitoring and logging are essential components of effective Node.js Deployments. By integrating tools that monitor application performance and log errors in real-time, you can quickly diagnose issues and maintain system stability.
Example: Integrating Winston Logging in a Node.js Application
Winston is a flexible logging library for Node.js that can be easily integrated into your application. Below is an example of how to set up Winston logging:
const winston = require('winston');
const logger = winston.createLogger({
level: 'info',
format: winston.format.combine(
winston.format.timestamp(),
winston.format.json()
),
transports: [
new winston.transports.Console(),
new winston.transports.File({ filename: 'combined.log' })
]
});
// Sample usage in an Express route
const express = require('express');
const app = express();
app.get('/', (req, res) => {
logger.info('Handling request for /');
res.send('Hello from Node.js Deployments with CI/CD Pipelines!');
});
app.listen(3000, () => {
logger.info('Server started on port 3000');
});
Explanation:
- Logger Creation: Winston is configured to log information-level messages with timestamps in JSON format.
- Transports: Logs are sent both to the console and a file (
combined.log
). - Usage: The logger is used within an Express route to record incoming requests.
Integrating such monitoring and logging tools in your Node.js Deployments enables you to track application health, quickly pinpoint issues, and maintain high performance. For more information on Winston, visit the Winston GitHub repository.
Master this concept through DevOps Tools in the Industry: A Comprehensive Guide to Mastering the Art of DevOps
Node.js Deployments: Security and Compliance Automation
Security is non-negotiable in modern Node.js Deployments. Automating security checks and compliance verifications within your CI/CD pipeline ensures that vulnerabilities are identified and addressed promptly. Tools like ESLint for code quality, Snyk for vulnerability scanning, and automated security tests are integral to this process.
Example: Integrating Snyk Security Scanning in CI/CD
Below is an example of how you can integrate Snyk into a GitHub Actions workflow to scan your Node.js application for vulnerabilities:
name: Security Scan with Snyk
on:
push:
branches: [ main ]
pull_request:
branches: [ main ]
jobs:
security:
runs-on: ubuntu-latest
steps:
- name: Checkout Code
uses: actions/checkout@v2
- name: Setup Node.js
uses: actions/setup-node@v2
with:
node-version: 16
- name: Install Dependencies
run: npm install
- name: Run Snyk Test
uses: snyk/actions/node@master
env:
SNYK_TOKEN: ${{ secrets.SNYK_TOKEN }}
Explanation:
- Snyk Integration: The workflow integrates Snyk to automatically scan for vulnerabilities each time new code is pushed.
- Automated Security Checks: If any issues are found, the pipeline can be configured to fail, preventing insecure code from being deployed.
Automating security checks as part of your Node.js Deployments pipeline is essential for maintaining a secure codebase and ensuring compliance with industry standards. For additional insights, review the Snyk documentation.
Delve into specifics at A Complete Guide to AWS Security Features: Protecting Your Cloud Infrastructure
Conclusion
Efficient Node.js Deployments with CI/CD Pipelines can transform your development process, enabling faster, more reliable, and secure application releases. In this guide, we’ve explored nine distinct methods—from automated testing integration and containerization with Docker to zero downtime releases, infrastructure as code, rolling updates, GitOps, monitoring and logging, and robust security practices. Each of these approaches not only streamlines deployment but also builds a resilient framework for future growth.
By integrating these strategies into your CI/CD pipelines, you ensure that every deployment is consistent, reproducible, and scalable. The coding examples and external resources provided throughout this guide serve as practical starting points to deepen your understanding and implement best practices in your projects. As you continue to refine your approach, always remember the importance of automated testing, secure configuration management, and continuous monitoring in your Node.js Deployments.
Moving forward, consider exploring advanced topics like microservices architecture and serverless deployments, which can further enhance your application’s performance and scalability. Embrace these techniques to build a robust, efficient, and secure deployment pipeline that meets the demands of modern software development.
For additional insights and up-to-date practices, revisit the resources linked in each section and join communities such as the Node.js community on GitHub and Docker forums. By continuously learning and adapting, you’ll be well-equipped to tackle new challenges and leverage the full potential of Node.js Deployments with CI/CD Pipelines.
In summary, this comprehensive guide has provided an in-depth exploration of nine ways to implement effective Node.js Deployments within CI/CD pipelines. Each method is designed to build upon your knowledge progressively while offering practical code examples, troubleshooting tips, and external resources to ensure your deployments are secure, efficient, and scalable. As you integrate these strategies into your development workflow, you’ll be better prepared to deliver high-quality applications with minimal downtime and maximum reliability.
Happy coding, and may your Node.js Deployments continually evolve to meet the ever-changing demands of modern software development!
Similar Articles
Related Content
More from devops
Explore how Jenkins versions have shaped modern CI/CD practices. This comprehensive guide traces the …
Learn essential Jenkins Versions strategies to optimize your CI/CD pipelines. This guide covers …
You Might Also Like
Discover 13 essential bash functions to handle time change, daylight savings 2025, spring forward …
Explore how OpenAI transforms development workflows, empowering developers and DevOps teams with …
Discover the best Linux automation tools like Ansible, Terraform, and Docker. Learn how to automate …
Contents
- Introduction
- Node.js Deployments: Automated Testing Integration
- Node.js Deployments: Containerization with Docker
- Node.js Deployments: Environment Variable Management
- Node.js Deployments: Zero Downtime Releases
- Node.js Deployments: Infrastructure as Code
- Node.js Deployments: Rolling Updates Strategy
- Node.js Deployments: GitOps for Automation
- Node.js Deployments: Monitoring and Logging Integration
- Node.js Deployments: Security and Compliance Automation
- Conclusion