Complete tutorial on deploying Jenkins to Amazon EKS. Learn what pods are, why deployments matter, …
OpenAI: Essential Benefits for Modern Development and DevOps - Complete Guide OpenAI: Essential Benefits for Modern Development and DevOps - Complete Guide
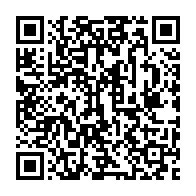
Summary
Understanding OpenAI’s Development Impact
OpenAI has fundamentally transformed how developers and DevOps professionals approach their daily tasks. By providing advanced AI models and tools, OpenAI addresses critical challenges in code development, testing, and deployment. The technology significantly reduces development time while improving code quality and consistency across projects.
The integration of OpenAI’s capabilities into development workflows has become increasingly crucial as organizations seek to maintain competitive advantages in rapidly evolving technical landscapes. From automated code generation to intelligent testing frameworks, OpenAI’s solutions provide the foundation for modern development practices.
Expand your knowledge with Node.js and LangChain: Bridging JavaScript and AI
OpenAI’s Core Benefits for Developers
OpenAI’s impact on development productivity manifests through various tools and capabilities that streamline the coding process. By leveraging OpenAI’s advanced models, developers can automate routine tasks and focus on more complex problem-solving.
Deepen your understanding in How DevOps Integrates with AI: Enhancing Automation and Efficiency in Software Development
# Example: Automated Documentation Generation using OpenAI
import openai
def generate_documentation(code_snippet):
try:
response = openai.ChatCompletion.create(
model="gpt-4",
messages=[
{"role": "system", "content": "Generate comprehensive documentation for the following code."},
{"role": "user", "content": f"Document this code:\n{code_snippet}"}
],
temperature=0.7
)
return response.choices[0].message.content
except Exception as e:
return f"Documentation generation failed: {str(e)}"
# Example usage
code = """
def process_data(data_list):
return [x * 2 for x in data_list if x > 0]
"""
documentation = generate_documentation(code)
print(documentation)
OpenAI’s DevOps Integration Advantages
DevOps teams benefit significantly from OpenAI’s automation capabilities, particularly in areas of deployment, monitoring, and maintenance. The technology enables intelligent system analysis and predictive maintenance, reducing downtime and improving operational efficiency.
Explore this further in Revolutionizing DevOps: The Impact of AI and Machine Learning
# Example: Automated Log Analysis with OpenAI
def analyze_system_logs(log_content):
try:
response = openai.ChatCompletion.create(
model="gpt-4",
messages=[
{"role": "system", "content": "Analyze system logs for potential issues and provide recommendations."},
{"role": "user", "content": f"Analyze these logs:\n{log_content}"}
],
max_tokens=500
)
return response.choices[0].message.content
except Exception as e:
return f"Log analysis failed: {str(e)}"
OpenAI’s Code Quality Enhancement Features
OpenAI improves code quality through automated review processes and intelligent suggestions. The technology helps identify potential bugs, security vulnerabilities, and performance issues before they reach production.
Discover related concepts in Revolutionizing DevOps: The Impact of AI and Machine Learning
# Example: Code Quality Check using OpenAI
def check_code_quality(code_snippet):
prompt = f"""
Analyze this code for:
1. Potential bugs
2. Security vulnerabilities
3. Performance optimizations
4. Best practice violations
Code:
{code_snippet}
"""
try:
response = openai.ChatCompletion.create(
model="gpt-4",
messages=[
{"role": "system", "content": "You are an expert code reviewer focused on quality and security."},
{"role": "user", "content": prompt}
]
)
return response.choices[0].message.content
except Exception as e:
return f"Code quality check failed: {str(e)}"
OpenAI’s Automated Testing Solutions
OpenAI enhances testing processes by generating test cases, identifying edge cases, and automating test suite creation. This capability ensures more comprehensive test coverage while reducing the time required for test development.
Uncover more details in Revolutionizing DevOps: The Impact of AI and Machine Learning
# Example: Automated Test Case Generation
def generate_test_cases(function_code):
try:
response = openai.ChatCompletion.create(
model="gpt-4",
messages=[
{"role": "system", "content": "Generate comprehensive unit tests for the given function."},
{"role": "user", "content": f"Create test cases for:\n{function_code}"}
]
)
return response.choices[0].message.content
except Exception as e:
return f"Test case generation failed: {str(e)}"
OpenAI’s Performance Optimization Capabilities
OpenAI assists in identifying and resolving performance bottlenecks through intelligent code analysis and optimization suggestions. This capability helps developers maintain high-performance applications while reducing resource consumption.
Journey deeper into this topic with Node.js and LangChain: Bridging JavaScript and AI
OpenAI’s Security Enhancement Features
Security benefits from OpenAI include automated vulnerability scanning, secure code generation, and intelligent threat detection. These features help maintain robust security practices throughout the development lifecycle.
Enrich your learning with A Complete Guide to AWS Security Features: Protecting Your Cloud Infrastructure
OpenAI’s Collaboration Improvements
OpenAI enhances team collaboration through intelligent code documentation, automated code review comments, and standardized coding practices. This leads to better knowledge sharing and maintained code quality across teams.
Gain comprehensive insights from Revolutionizing DevOps: The Impact of AI and Machine Learning
OpenAI’s Cost and Time Savings
The implementation of OpenAI solutions results in significant cost and time savings through:
Master this concept through Revolutionizing DevOps: The Impact of AI and Machine Learning
- Reduced development time
- Faster bug detection and resolution
- Automated routine tasks
- Improved code maintenance
- Enhanced deployment efficiency
OpenAI’s Integration Best Practices
To maximize the benefits of OpenAI in development workflows, organizations should follow these integration practices:
Delve into specifics at OpenAI Node.js Integration: Complete Developer's Guide [2024]
- Establish clear usage guidelines
- Implement proper API security measures
- Monitor and optimize API usage
- Maintain updated dependencies
- Provide team training on OpenAI tools
Conclusion: OpenAI’s Development Impact
OpenAI continues to revolutionize software development and DevOps practices, providing essential tools that enhance productivity, quality, and efficiency. As organizations increasingly adopt OpenAI’s capabilities, the technology becomes more integral to modern development workflows, offering compelling benefits that drive innovation and success in software development.
External Resources:
- OpenAI Documentation: https://platform.openai.com/docs
- OpenAI for Developers: https://openai.com/blog/openai-api
- OpenAI GitHub: https://github.com/openai
- OpenAI API Reference: https://platform.openai.com/docs/api-reference
Similar Articles
Related Content
More from devops
Explore how Jenkins versions have shaped modern CI/CD practices. This comprehensive guide traces the …
Learn essential Jenkins Versions strategies to optimize your CI/CD pipelines. This guide covers …
You Might Also Like
Explore 9 innovative methods for Node.js deployments using CI/CD pipelines. Learn how to automate, …
Master Jenkinsfile with envsubst to streamline your CI/CD pipelines. Learn how environment variable …
Explore essential AWS security features that protect your cloud infrastructure from threats. Learn …
Contents
- Understanding OpenAI’s Development Impact
- OpenAI’s Core Benefits for Developers
- OpenAI’s DevOps Integration Advantages
- OpenAI’s Code Quality Enhancement Features
- OpenAI’s Automated Testing Solutions
- OpenAI’s Performance Optimization Capabilities
- OpenAI’s Security Enhancement Features
- OpenAI’s Collaboration Improvements
- OpenAI’s Cost and Time Savings
- OpenAI’s Integration Best Practices
- Conclusion: OpenAI’s Development Impact