Complete tutorial on deploying Jenkins to Amazon EKS. Learn what pods are, why deployments matter, …
Self-Healing Bash: Creating Resilient Functions That Recover From Failures Self-Healing Bash: Creating Resilient Functions That Recover From Failures
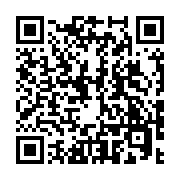
Summary
A few months ago, our monitoring system alerted me at 2 AM about a critical ETL pipeline failure. Half-awake, I logged in to find our Bash script had crashed because an API endpoint was temporarily down. The script made no attempt to retry the connection, fix the issue, or gracefully degrade. Instead, it simply died—leaving our data pipeline broken until I manually intervened.
That night sparked my journey into creating self-healing Bash functions. After studying resources like “Linux System Programming: Talking Directly to the Kernel and C Library” by Robert Love and implementing principles from “How Linux Works” by Brian Ward, I’ve transformed our brittle scripts into resilient automation that can detect, troubleshoot, and often recover from failures without human intervention.
What Makes Bash Functions Self-Healing?
Self-healing Bash functions are designed to automatically recover from failures rather than simply reporting them. According to principles outlined in “linux-bash-and-more/P200000002838/9781118024485">Shell Scripting” guides, truly resilient functions share these key characteristics:
- They detect failures quickly and accurately
- They implement strategic retries for transient failures
- They can repair their own environment
- They have fallback mechanisms when primary methods fail
- They maintain state between recovery attempts
- They know when to escalate vs. when to self-recover
As Robert Love explains in “Linux System Programming”, this approach shifts our perspective from “fail and report” to “detect and repair”—a fundamental difference in how we think about script reliability.
The flow of a self-healing function looks like this:
Expand your knowledge with Advanced String Operations in Bash: Building Custom Functions
[Function Called]
|
v
[Attempt Operation] --> [Operation Succeeds] --> [Return Success]
|
v
[Operation Fails]
|
v
[Diagnose Failure] --> [Is it recoverable?] --> [No] --> [Fail Gracefully]
| |
v v
[Yes, Known Issue] [Escalate/Alert]
|
v
[Apply Recovery Strategy]
|
v
[Retry Operation] --> [Success?] --> [Yes] --> [Return Success]
| |
v v
[Max Retries?] --> [No] --> [Adjust Strategy]
|
v
[Yes]
|
v
[Fallback Method] --> [Success?] --> [Yes] --> [Return With Warning]
| |
v v
[No] [Log Success]
|
v
[Fail Gracefully]
Building Basic Self-Healing Bash Functions
Let’s start with the fundamentals of self-healing Bash functions. The principles I’ve learned from “Unix Power Tools” emphasize starting with a solid foundation before adding complexity.
1. Implement Retry Logic for Transient Failures
Here’s a basic retry function that forms the foundation of self-healing:
#!/bin/bash
function retry_command {
local -r cmd="$1"
local -r max_attempts="${2:-3}"
local -r delay="${3:-5}"
local -r error_msg="${4:-Command failed}"
# Initialize counter
local attempt=1
# Try the command up to max_attempts times
until $cmd; do
# Check if we've reached max attempts
if ((attempt >= max_attempts)); then
echo "ERROR: $error_msg after $max_attempts attempts" >&2
return 1
fi
# Log retry attempt
echo "WARNING: $error_msg (attempt $attempt/$max_attempts)" >&2
echo "Retrying in $delay seconds..." >&2
# Wait before retry
sleep "$delay"
# Increment counter
((attempt++))
done
# If we get here, the command succeeded
if ((attempt > 1)); then
echo "SUCCESS: Command succeeded after $((attempt-1)) retries" >&2
fi
return 0
}
# Example usage
retry_command "curl -s https://api.example.com/endpoint > data.json" 5 10 "API request failed"
This function provides basic resilience against transient failures like network connectivity issues. According to “How Linux Works”, most network-related failures are temporary and can be resolved with simple retries.
2. Add Progressive Backoff for Resource-Intensive Operations
Let’s enhance our retry logic with progressive backoff:
#!/bin/bash
function retry_with_backoff {
local -r cmd="$1"
local -r max_attempts="${2:-5}"
local -r initial_delay="${3:-2}"
local -r max_delay="${4:-60}"
local -r error_msg="${5:-Command failed}"
local attempt=1
local delay="$initial_delay"
until $cmd; do
local exit_code=$?
if ((attempt >= max_attempts)); then
echo "ERROR: $error_msg after $max_attempts attempts (exit code: $exit_code)" >&2
return $exit_code
fi
echo "WARNING: $error_msg (attempt $attempt/$max_attempts, exit code: $exit_code)" >&2
echo "Retrying in $delay seconds..." >&2
sleep "$delay"
# Calculate next delay (exponential backoff with jitter)
delay=$(( delay * 2 + (RANDOM % 5) ))
# Cap the delay at max_delay
if ((delay > max_delay)); then
delay="$max_delay"
fi
((attempt++))
done
if ((attempt > 1)); then
echo "SUCCESS: Command succeeded after $((attempt-1)) retries" >&2
fi
return 0
}
# Example usage
retry_with_backoff "ssh server.example.com 'df -h'" 5 2 60 "Connection to server failed"
This improved version implements exponential backoff with a small random jitter, which is ideal for preventing thundering herd problems when multiple scripts are retrying simultaneously. “Shell Scripting” guides recommend this approach for API calls and resource-intensive operations.
Deepen your understanding in Advanced String Operations in Bash: Building Custom Functions
Creating Self-Diagnosing Bash Functions
The next level of self-healing involves functions that can diagnose what went wrong and adapt their recovery strategy accordingly. Brian Ward’s “How Linux Works” emphasizes the importance of understanding failure modes to implement effective recovery strategies.
1. Database Connection Function with Self-Diagnostics
#!/bin/bash
function connect_database {
local -r db_host="$1"
local -r db_name="$2"
local -r db_user="$3"
local -r db_pass="$4"
local -r max_attempts=5
local attempt=1
# Function to test database connection
function test_connection {
PGPASSWORD="$db_pass" psql -h "$db_host" -U "$db_user" -d "$db_name" -c "SELECT 1" &>/dev/null
}
# Try to connect
until test_connection; do
local exit_code=$?
# Max attempts reached
if ((attempt >= max_attempts)); then
echo "ERROR: Failed to connect to database after $max_attempts attempts" >&2
return 1
fi
# Diagnose the issue based on the error code
case $exit_code in
1)
echo "Connection refused. Checking if PostgreSQL service is running..." >&2
if ssh "$db_host" "systemctl is-active postgresql" &>/dev/null; then
echo "PostgreSQL service is running. Waiting for it to accept connections..." >&2
else
echo "PostgreSQL service is down. Attempting to start it..." >&2
ssh "$db_host" "sudo systemctl start postgresql" || {
echo "ERROR: Failed to start PostgreSQL service" >&2
return 2
}
echo "PostgreSQL service started. Waiting for it to initialize..." >&2
sleep 10
fi
;;
2)
echo "Authentication failed. Checking credentials..." >&2
if [[ -f ~/.pgpass_backup ]]; then
echo "Restoring backup credentials..." >&2
cp ~/.pgpass_backup ~/.pgpass
chmod 600 ~/.pgpass
fi
;;
3)
echo "Database $db_name does not exist. Checking if we can create it..." >&2
if PGPASSWORD="$db_pass" psql -h "$db_host" -U "$db_user" -d "postgres" -c "CREATE DATABASE $db_name;" &>/dev/null; then
echo "Created database $db_name" >&2
return 0
else
echo "ERROR: Could not create database $db_name" >&2
fi
;;
*)
echo "Unknown error (code $exit_code). Waiting before retry..." >&2
;;
esac
sleep $((attempt * 2))
((attempt++))
done
if ((attempt > 1)); then
echo "SUCCESS: Connected to database after $((attempt-1)) retries" >&2
else
echo "SUCCESS: Connected to database" >&2
fi
# Backup working credentials
if [[ -f ~/.pgpass && ! -f ~/.pgpass_backup ]]; then
cp ~/.pgpass ~/.pgpass_backup
fi
return 0
}
# Example usage
connect_database "db.example.com" "analytics" "datauser" "password123"
This function not only retries connections but actively diagnoses different failure modes and takes specific recovery actions for each one. Robert Love’s “Linux System Programming” highlights this type of contextualized error handling as a key difference between amateur and professional automation.
2. Self-Healing Disk Space Management
Here’s a practical example of a function that monitors and remediates disk space issues:
#!/bin/bash
function ensure_disk_space {
local -r directory="$1"
local -r required_mb="${2:-100}"
local -r cleanup_threshold_mb="${3:-500}"
# Get available disk space in MB
local available_mb
available_mb=$(df -m --output=avail "$(readlink -f "$directory")" | tail -1 | tr -d ' ')
echo "Available disk space: $available_mb MB (need $required_mb MB)" >&2
# Check if we have enough space
if ((available_mb >= required_mb)); then
return 0
fi
echo "WARNING: Low disk space. Need $required_mb MB but only have $available_mb MB" >&2
echo "Attempting to free up disk space..." >&2
# Strategy 1: Clean up temp files
echo "Cleaning up temporary files..." >&2
find /tmp -type f -atime +7 -delete
# Strategy 2: Clear package cache if we're on a system with apt
if command -v apt-get &>/dev/null; then
echo "Cleaning apt cache..." >&2
apt-get clean
fi
# Strategy 3: Clean up old logs
echo "Compressing logs older than 7 days..." >&2
find /var/log -type f -name "*.log" -mtime +7 -exec gzip -9 {} \; 2>/dev/null || true
# Strategy 4: Remove old Docker images if Docker is installed
if command -v docker &>/dev/null; then
echo "Removing unused Docker images..." >&2
docker system prune -f
fi
# Check available space again
available_mb=$(df -m --output=avail "$(readlink -f "$directory")" | tail -1 | tr -d ' ')
echo "Available disk space after cleanup: $available_mb MB" >&2
# If we still don't have enough space, try more aggressive cleanup
if ((available_mb < required_mb)); then
echo "WARNING: Still insufficient disk space. Trying more aggressive cleanup..." >&2
# Find and remove the largest files in /tmp
echo "Removing largest files in /tmp..." >&2
find /tmp -type f -size +50M -delete
# Remove old journal logs
if command -v journalctl &>/dev/null; then
echo "Clearing journal logs..." >&2
journalctl --vacuum-time=3d
fi
# Check space again
available_mb=$(df -m --output=avail "$(readlink -f "$directory")" | tail -1 | tr -d ' ')
echo "Available disk space after aggressive cleanup: $available_mb MB" >&2
if ((available_mb < required_mb)); then
echo "ERROR: Failed to free up enough disk space" >&2
return 1
fi
fi
echo "SUCCESS: Freed up disk space. Now have $available_mb MB available" >&2
return 0
}
# Example usage: ensure we have at least 200MB of space in the current directory
ensure_disk_space "." 200
This function shows how self-healing Bash can progressively apply more aggressive remediation strategies until the issue is resolved. “Unix Power Tools” recommends this layered approach to self-healing, starting with safe, non-destructive fixes before moving to more impactful solutions.
Explore this further in Advanced String Operations in Bash: Building Custom Functions
Implementing Environment Self-Repair in Bash
Another aspect of self-healing Bash functions is the ability to repair the environment they operate in. Brian Ward’s “How Linux Works” provides insights into how Linux environments can be programmatically repaired.
1. Self-Healing Dependency Checker and Installer
#!/bin/bash
function ensure_dependencies {
local -r dependencies=("$@")
local missing_deps=()
# Check for each dependency
for dep in "${dependencies[@]}"; do
if ! command -v "$dep" &>/dev/null; then
missing_deps+=("$dep")
echo "Missing dependency: $dep" >&2
fi
done
# If no missing dependencies, we're good
if [ ${#missing_deps[@]} -eq 0 ]; then
echo "All dependencies are installed" >&2
return 0
fi
echo "Attempting to install missing dependencies: ${missing_deps[*]}" >&2
# Detect package manager
if command -v apt-get &>/dev/null; then
PM="apt-get"
PM_INSTALL="apt-get install -y"
PM_UPDATE="apt-get update"
elif command -v yum &>/dev/null; then
PM="yum"
PM_INSTALL="yum install -y"
PM_UPDATE="yum check-update"
elif command -v brew &>/dev/null; then
PM="brew"
PM_INSTALL="brew install"
PM_UPDATE="brew update"
else
echo "ERROR: Could not detect package manager. Please install: ${missing_deps[*]}" >&2
return 1
fi
# Update package list
echo "Updating package lists..." >&2
$PM_UPDATE
# Map common command names to package names (extend as needed)
declare -A pkg_map
pkg_map[curl]="curl"
pkg_map[wget]="wget"
pkg_map[jq]="jq"
pkg_map[aws]="awscli"
pkg_map[docker]="docker"
pkg_map[git]="git"
pkg_map[python]="python"
pkg_map[python3]="python3"
pkg_map[node]="nodejs"
pkg_map[npm]="npm"
# Install each missing dependency
for dep in "${missing_deps[@]}"; do
local pkg="${pkg_map[$dep]:-$dep}"
echo "Installing $pkg..." >&2
if $PM_INSTALL "$pkg"; then
echo "Successfully installed $pkg" >&2
else
echo "ERROR: Failed to install $pkg" >&2
return 1
fi
done
echo "All dependencies have been installed" >&2
return 0
}
# Example usage
ensure_dependencies curl jq aws git
This function not only identifies missing dependencies but also attempts to install them using the appropriate package manager for the system. According to principles outlined in “Shell Scripting” guides, this type of environment self-repair is critical for scripts that need to run in diverse environments.
2. Config File Self-Healing
Here’s a function that ensures a configuration file exists and has all required settings:
#!/bin/bash
function ensure_config {
local -r config_file="$1"
local -r default_config="$2"
local -r required_keys=("${@:3}")
# Check if config file exists
if [[ ! -f "$config_file" ]]; then
echo "Config file $config_file does not exist. Creating it..." >&2
# Create directory if it doesn't exist
mkdir -p "$(dirname "$config_file")"
# Copy default config if provided
if [[ -n "$default_config" && -f "$default_config" ]]; then
cp "$default_config" "$config_file"
echo "Copied default configuration from $default_config" >&2
else
# Create empty config file
touch "$config_file"
echo "Created empty configuration file" >&2
fi
fi
# Check file permissions
if [[ ! -r "$config_file" ]]; then
echo "Config file $config_file is not readable. Fixing permissions..." >&2
chmod 644 "$config_file"
fi
# Check for required keys
local missing_keys=()
for key in "${required_keys[@]}"; do
if ! grep -q "^$key=" "$config_file"; then
missing_keys+=("$key")
fi
done
# Add missing keys with default values
if [[ ${#missing_keys[@]} -gt 0 ]]; then
echo "Adding missing configuration keys: ${missing_keys[*]}" >&2
for key in "${missing_keys[@]}"; do
case "$key" in
"api_url")
echo "api_url=https://api.example.com/v1" >> "$config_file"
;;
"timeout")
echo "timeout=30" >> "$config_file"
;;
"retry_count")
echo "retry_count=3" >> "$config_file"
;;
"log_level")
echo "log_level=info" >> "$config_file"
;;
*)
echo "$key=PLACEHOLDER_CHANGE_ME" >> "$config_file"
;;
esac
done
echo "Configuration file has been updated with defaults for missing keys" >&2
fi
echo "Configuration file $config_file is valid and contains all required keys" >&2
return 0
}
# Example usage
ensure_config "/etc/myapp/config.ini" "/etc/myapp/default_config.ini" "api_url" "timeout" "retry_count" "log_level"
This function demonstrates how Bash can ensure that configuration files not only exist but contain all required settings. As Robert Love explains in “Linux System Programming”, configuration validation is a common failure point that can be effectively self-healed.
Discover related concepts in Bash for SRE: Implementing Google's Reliability Engineering Principles in Shell Scripts
Advanced Self-Healing: Stateful Recovery and Checkpoints
For longer-running Bash functions, we need ways to save state and resume operations after failures. “Shell Scripting” guides recommend implementing checkpoints for resilient processing of large datasets.
1. Resumable File Processing
#!/bin/bash
function process_files_with_resume {
local -r source_dir="$1"
local -r pattern="$2"
local -r checkpoint_file="$3"
# Create checkpoint file if it doesn't exist
if [[ ! -f "$checkpoint_file" ]]; then
touch "$checkpoint_file"
fi
# Find all matching files
local files
mapfile -t files < <(find "$source_dir" -type f -name "$pattern")
echo "Found ${#files[@]} files to process" >&2
# Determine which files have already been processed
local processed_count=0
for file in "${files[@]}"; do
if grep -q "^$(basename "$file")$" "$checkpoint_file"; then
echo "Skipping already processed file: $file" >&2
((processed_count++))
continue
fi
echo "Processing file: $file" >&2
# Here's where you'd put your actual file processing logic
# For example:
if process_single_file "$file"; then
# Record successful processing
basename "$file" >> "$checkpoint_file"
echo "Successfully processed file: $file" >&2
else
echo "ERROR: Failed to process file: $file" >&2
# Optionally: return failure here, or continue with other files
# For this example, we'll continue with other files
fi
done
echo "Processing complete. $processed_count files were already processed and ${#files[@]} total files were found." >&2
return 0
}
# Example file processing function
function process_single_file {
local file="$1"
# Simulate processing with random success/failure
if (( RANDOM % 10 != 0 )); then
# 90% success rate for demonstration
sleep 1
return 0
else
# 10% failure rate for demonstration
return 1
fi
}
# Example usage
process_files_with_resume "/data/logs" "*.log" "/tmp/processed_logs.checkpoint"
This function demonstrates how to implement checkpointing for processing large sets of files. According to “Unix Power Tools”, this pattern is essential for making Bash scripts robust against interruptions and partial failures.
2. Transaction-Like Processing for Multiple Steps
Here’s a more complex example that implements a transaction-like pattern with rollback capability:
#!/bin/bash
function deploy_with_rollback {
local -r app_name="$1"
local -r version="$2"
local -r deploy_dir="$3"
local -r state_dir="${4:-/var/lib/deployments/$app_name}"
# Ensure state directory exists
mkdir -p "$state_dir"
# Track what steps have been completed
local state_file="$state_dir/deploy_${version}.state"
touch "$state_file"
# Define the deployment steps
local -a steps=(
"backup"
"download"
"stop_service"
"install"
"configure"
"start_service"
"verify"
)
# Define rollback functions
function rollback_backup {
echo "No rollback needed for backup step" >&2
}
function rollback_download {
echo "Removing downloaded package..." >&2
rm -f "$deploy_dir/$app_name-$version.tar.gz"
}
function rollback_stop_service {
echo "Restarting service..." >&2
systemctl start "$app_name" || true
}
function rollback_install {
echo "Removing installed files..." >&2
rm -rf "$deploy_dir/$app_name-$version"
# Restore previous version if available
if [[ -d "$deploy_dir/$app_name-previous" ]]; then
mv "$deploy_dir/$app_name-previous" "$deploy_dir/$app_name"
fi
}
function rollback_configure {
echo "Restoring previous configuration..." >&2
if [[ -f "$deploy_dir/config/$app_name.conf.bak" ]]; then
mv "$deploy_dir/config/$app_name.conf.bak" "$deploy_dir/config/$app_name.conf"
fi
}
function rollback_start_service {
echo "Stopping newly started service..." >&2
systemctl stop "$app_name" || true
# If we had a previous version running, restart it
if grep -q "stop_service" "$state_file"; then
echo "Restarting previous version..." >&2
rollback_install
rollback_configure
systemctl start "$app_name" || true
fi
}
function rollback_verify {
echo "No rollback needed for verification step" >&2
}
# Check which steps have already been completed
local start_step=0
for ((i=0; i<${#steps[@]}; i++)); do
if grep -q "${steps[$i]}" "$state_file"; then
start_step=$((i+1))
fi
done
echo "Starting deployment from step $((start_step+1)) (${steps[$start_step]})" >&2
# Execute remaining steps
for ((i=start_step; i<${#steps[@]}; i++)); do
local step="${steps[$i]}"
echo "Executing step: $step" >&2
# Execute the appropriate step
case "$step" in
backup)
echo "Creating backup..." >&2
if [[ -d "$deploy_dir/$app_name" ]]; then
cp -a "$deploy_dir/$app_name" "$deploy_dir/$app_name-backup-$version"
echo "backup" >> "$state_file"
else
echo "No existing installation to backup" >&2
echo "backup" >> "$state_file"
fi
;;
download)
echo "Downloading package..." >&2
if curl -s -o "$deploy_dir/$app_name-$version.tar.gz" "https://example.com/packages/$app_name-$version.tar.gz"; then
echo "download" >> "$state_file"
else
echo "ERROR: Failed to download package" >&2
rollback_download
return 1
fi
;;
stop_service)
echo "Stopping service..." >&2
if systemctl is-active "$app_name" &>/dev/null; then
if systemctl stop "$app_name"; then
echo "stop_service" >> "$state_file"
else
echo "ERROR: Failed to stop service" >&2
rollback_stop_service
return 1
fi
else
echo "Service not running" >&2
echo "stop_service" >> "$state_file"
fi
;;
install)
echo "Installing new version..." >&2
# Backup current version if exists
if [[ -d "$deploy_dir/$app_name" ]]; then
mv "$deploy_dir/$app_name" "$deploy_dir/$app_name-previous"
fi
# Extract new version
mkdir -p "$deploy_dir/$app_name-$version"
if tar -xzf "$deploy_dir/$app_name-$version.tar.gz" -C "$deploy_dir/$app_name-$version"; then
ln -sfn "$app_name-$version" "$deploy_dir/$app_name"
echo "install" >> "$state_file"
else
echo "ERROR: Failed to extract package" >&2
rollback_install
return 1
fi
;;
configure)
echo "Configuring application..." >&2
# Backup existing config if it exists
if [[ -f "$deploy_dir/config/$app_name.conf" ]]; then
cp "$deploy_dir/config/$app_name.conf" "$deploy_dir/config/$app_name.conf.bak"
fi
# Apply new config
mkdir -p "$deploy_dir/config"
if cp "$deploy_dir/$app_name/config/default.conf" "$deploy_dir/config/$app_name.conf"; then
echo "configure" >> "$state_file"
else
echo "ERROR: Failed to configure application" >&2
rollback_configure
return 1
fi
;;
start_service)
echo "Starting service..." >&2
if systemctl start "$app_name"; then
echo "start_service" >> "$state_file"
else
echo "ERROR: Failed to start service" >&2
rollback_start_service
return 1
fi
;;
verify)
echo "Verifying deployment..." >&2
# Wait for service to fully initialize
sleep 5
# Check if service is responding
if curl -s "http://localhost:8080/$app_name/health" | grep -q "ok"; then
echo "verify" >> "$state_file"
echo "SUCCESS: Deployment completed successfully" >&2
else
echo "ERROR: Service verification failed" >&2
rollback_start_service
return 1
fi
;;
esac
done
# Clean up old backups and state files
find "$deploy_dir" -name "$app_name-backup-*" -mtime +7 -exec rm -rf {} \; 2>/dev/null || true
find "$state_dir" -name "deploy_*.state" -mtime +7 -exec rm -f {} \; 2>/dev/null || true
echo "Deployment of $app_name version $version completed successfully" >&2
return 0
}
# Example usage
deploy_with_rollback "myapp" "1.2.3" "/opt/applications"
This complex function demonstrates several advanced self-healing principles:
- State tracking to allow resuming from the last successful step
- Step-by-step progression with verification at each stage
- Rollback capabilities for each step if something goes wrong
- Cleanup of old state files and backups
According to principles in Brian Ward’s “How Linux Works”, this transaction-like approach is essential for complex operations where partial completion can leave systems in an inconsistent state.
Uncover more details in Advanced Bash Scripting Techniques for Automation: A Comprehensive Guide
Building Real-World Self-Healing Pipelines with Bash
Combining the techniques we’ve covered allows us to build complete self-healing pipelines. Here’s a practical example of a data processing pipeline with multiple self-healing capabilities:
#!/bin/bash
# Set strict mode
set -uo pipefail
# Global configuration
CONFIG_FILE="/etc/data-pipeline/config.ini"
LOG_FILE="/var/log/data-pipeline.log"
CHECKPOINT_FILE="/var/lib/data-pipeline/checkpoint.dat"
MAX_RETRIES=5
BACKOFF_TIME=10
# Load helper functions (assuming they're defined in external files)
source "$(dirname "$0")/lib/retry.sh"
source "$(dirname "$0")/lib/logging.sh"
source "$(dirname "$0")/lib/config.sh"
function log {
local -r level="$1"
local -r message="$2"
echo "[$(date '+%Y-%m-%d %H:%M:%S')] [$level] $message" | tee -a "$LOG_FILE"
}
function ensure_environment {
# Ensure config exists and has required settings
ensure_config "$CONFIG_FILE" "/etc/data-pipeline/default_config.ini" \
"source_dir" "destination_dir" "processing_threads" "archive_days" "api_key"
# Load configuration
source "$CONFIG_FILE"
# Ensure required tools are installed
ensure_dependencies jq curl aws parallel
# Ensure directories exist
mkdir -p "$source_dir" "$destination_dir" "$(dirname "$LOG_FILE")" "$(dirname "$CHECKPOINT_FILE")"
# Ensure adequate disk space
ensure_disk_space "$destination_dir" 1000
# Ensure database connection (if needed)
if [[ -n "${db_host:-}" ]]; then
connect_database "$db_host" "$db_name" "$db_user" "$db_pass"
fi
log "INFO" "Environment setup completed successfully"
return 0
}
function fetch_data {
local -r api_url="$1"
local -r api_key="$2"
local -r output_file="$3"
log "INFO" "Fetching data from API..."
# Use retry with backoff for API calls
if retry_with_backoff "curl -s -H 'Authorization: Bearer $api_key' '$api_url' > '$output_file'" 3 5 60 "API fetch failed"; then
# Validate the response
if jq empty "$output_file" 2>/dev/null; then
log "INFO" "Successfully fetched and validated data"
return 0
else
log "ERROR" "API returned invalid JSON"
return 1
fi
else
log "ERROR" "Failed to fetch data after multiple retries"
return 1
fi
}
function process_data_file {
local -r input_file="$1"
local -r output_dir="$2"
local -r file_name="$(basename "$input_file")"
local -r output_file="$output_dir/${file_name%.json}_processed.csv"
log "INFO" "Processing file: $file_name"
# Check if this file was already processed
if grep -q "^$file_name$" "$CHECKPOINT_FILE"; then
log "INFO" "Skipping already processed file: $file_name"
return 0
fi
# Process the data with jq and convert to CSV
if jq -r '.data[] | [.id, .name, .value] | @csv' "$input_file" > "$output_file"; then
# Record that this file was processed
echo "$file_name" >> "$CHECKPOINT_FILE"
log "INFO" "Successfully processed file: $file_name"
return 0
else
log "ERROR" "Failed to process file: $file_name"
return 1
fi
}
function upload_to_s3 {
local -r source_file="$1"
local -r bucket="$2"
local -r prefix="$3"
local -r file_name="$(basename "$source_file")"
log "INFO" "Uploading file to S3: $file_name"
# Try to upload with retry logic
if retry_with_backoff "aws s3 cp '$source_file' 's3://$bucket/$prefix/$file_name'" 3 5 30 "S3 upload failed"; then
log "INFO" "Successfully uploaded file to S3: $file_name"
return 0
else
log "ERROR" "Failed to upload file to S3 after multiple retries"
return 1
fi
}
function run_pipeline {
log "INFO" "Starting data processing pipeline"
# Ensure environment is properly set up
ensure_environment || {
log "FATAL" "Failed to set up environment"
return 1
}
# Load configuration
source "$CONFIG_FILE"
# Create temp directory for today's data
local today_dir="$source_dir/$(date '+%Y%m%d')"
mkdir -p "$today_dir"
# Fetch fresh data
fetch_data "$api_url" "$api_key" "$today_dir/data_$(date '+%H%M%S').json" || {
log "ERROR" "Failed to fetch data, checking if we have previous data to process"
# Continue execution to process any existing files
}
# Find all data files and process them
log "INFO" "Processing all data files..."
local success_count=0
local failure_count=0
# Process each file
while IFS= read -r file; do
if process_data_file "$file" "$destination_dir"; then
((success_count++))
# Upload processed file to S3 if configured
if [[ -n "${s3_bucket:-}" ]]; then
local processed_file="${destination_dir}/$(basename "${file%.json}_processed.csv")"
upload_to_s3 "$processed_file" "$s3_bucket" "$s3_prefix" || {
log "WARN" "Failed to upload processed file to S3: $processed_file"
}
fi
else
((failure_count++))
fi
done < <(find "$source_dir" -type f -name "*.json" -mtime -1)
# Report results
log "INFO" "Pipeline completed: $success_count files processed successfully, $failure_count failures"
# Archive old files
if [[ -n "${archive_days:-}" && "${archive_days:-0}" -gt 0 ]]; then
log "INFO" "Archiving files older than $archive_days days"
find "$source_dir" -type f -mtime +"$archive_days" -exec mv {} "$source_dir/archive/" \;
fi
# Return success if at least some files were processed
if ((success_count > 0)); then
return 0
else
return 1
fi
}
# Run the pipeline
run_pipeline
This comprehensive example shows how multiple self-healing functions combine to create a robust pipeline that can:
- Set up its environment and dependencies
- Handle transient API failures
- Skip already processed files for idempotent operation
- Recover from partial executions
- Validate inputs and outputs
- Fall back to processing existing data if new data can’t be fetched
According to the principles in “Shell Scripting” and “Unix Power Tools”, this layered approach to self-healing is what makes Bash scripts reliable in production environments.
Journey deeper into this topic with Advanced String Operations in Bash: Building Custom Functions
Best Practices for Writing Self-Healing Bash Functions
Based on my experience and the principles in “Linux System Programming” by Robert Love, “How Linux Works” by Brian Ward, and “Unix Power Tools”, here are the best practices for creating self-healing Bash functions:
Always validate inputs and environment: Check prerequisites before starting work.
Use set flags: Set proper error handling flags like
set -euo pipefail
.Implement proper logging: Include timestamps, severity levels, and context.
Save state for resumability: Use checkpoint files to track progress.
Implement staged recovery: Try simple fixes first, then progressively more aggressive solutions.
Provide cleanup functions: Use
trap
to ensure proper cleanup even after failures.Add timeouts to all external calls: Prevent hanging on unresponsive services.
Define clear success/failure criteria: Know exactly what constitutes success.
Return meaningful exit codes: Different errors should return different exit codes.
Document recovery strategies: Comment your code to explain recovery logic.
Test failure scenarios: Deliberately break things to verify self-healing works.
Monitor recovery attempts: Log when self-healing kicks in for future analysis.
I’ve seen these practices transform brittle shell scripts into reliable automation that can run unattended for months. As “Shell Scripting” guides emphasize, the most reliable systems aren’t those that never fail—they’re the ones that can recover from failure without human intervention.
Enrich your learning with Advanced String Operations in Bash: Building Custom Functions
Conclusion: The Path to Bulletproof Bash Scripts
Creating self-healing Bash functions has transformed how I approach automation. The 2 AM wake-up calls have become increasingly rare, and when issues do occur, our scripts often resolve them before they become critical.
By implementing the techniques covered in this article—from basic retry logic to advanced stateful recovery—you can transform brittle Bash scripts into resilient automation tools that recover from failures automatically. As Brian Ward notes in “How Linux Works”, this shift from “monitor and alert” to “detect and repair” is fundamental to modern infrastructure automation.
The resources mentioned throughout this article—“Linux System Programming: Talking Directly to the Kernel and C Library” by Robert Love, “Unix Power Tools”, and “How Linux Works: What Every Superuser Should Know” by Brian Ward—provide deeper insights into the underlying principles. I encourage you to study them to develop a more profound understanding of how Linux systems can be programmed to heal themselves.
Remember that self-healing isn’t a single technique but a mindset—a commitment to designing automation that gracefully handles the messy, unpredictable nature of production environments. By embracing this mindset and implementing the patterns we’ve discussed, you’ll create Bash functions that don’t just automate tasks but adapt, learn, and recover when things inevitably go wrong.
What self-healing techniques have you implemented in your Bash scripts? I’d love to hear your stories and additional strategies in the comments below!
Similar Articles
Related Content
More from devops
Explore how Jenkins versions have shaped modern CI/CD practices. This comprehensive guide traces the …
Learn essential Jenkins Versions strategies to optimize your CI/CD pipelines. This guide covers …
You Might Also Like
Explore the fascinating history of the ls command from its Unix origins to modern Linux …
Master the Linux ls command with this comprehensive guide covering basic and advanced options, …
Master the Linux ls command with our task-oriented approach covering everyday file management …
Knowledge Quiz
Test your general knowledge with this quick quiz!
The quiz consists of 5 multiple-choice questions.
Take as much time as you need.
Your score will be shown at the end.
Question 1 of 5
Quiz Complete!
Your score: 0 out of 5
Loading next question...
Contents
- What Makes Bash Functions Self-Healing?
- Building Basic Self-Healing Bash Functions
- Creating Self-Diagnosing Bash Functions
- Implementing Environment Self-Repair in Bash
- Advanced Self-Healing: Stateful Recovery and Checkpoints
- Building Real-World Self-Healing Pipelines with Bash
- Best Practices for Writing Self-Healing Bash Functions
- Conclusion: The Path to Bulletproof Bash Scripts