Explore the fascinating history of the ls command from its Unix origins to modern Linux …
Building a YouTube-like System the Simple Way: AWS Lambda and S3 Building a YouTube-like System the Simple Way: AWS Lambda and S3
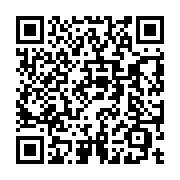
Summary
Building a YouTube-like System: The Simple Approach
I love simple solutions to complex problems. When I first needed to build a video platform similar to YouTube, I imagined months of work and complicated infrastructure. Then I discovered how AWS Lambda and S3 work together, and everything changed. This straightforward approach let me build a complete video platform in days, not months.
Let me walk you through how to build a YouTube-like system using AWS services. This approach is refreshingly simple - videos go in, get processed automatically, and come out ready for viewing. No complicated orchestration required! According to AWS’s documentation, this pattern can scale to handle millions of videos, so you can start small and grow without changing your architecture. The folks at A Cloud Guru call this the “serverless transformation” because it completely changes how we build and scale applications.
Expand your knowledge with Why You Need SQS in Your YouTube-like System: Beyond Basic Architecture
System Overview: The Building Blocks
Our YouTube-like system uses these key AWS components:
- S3 buckets for storing videos (both raw uploads and processed versions)
- Lambda functions that respond to events and coordinate processing
- MediaConvert for transcoding videos into different formats
- CloudFront for delivering videos globally
- DynamoDB for storing video metadata
- OpenSearch for making videos searchable
- Cognito for user management
I’ve named these resources to make them easy to understand:
raw-video-bucket
- Where uploaded videos first landprocessed-video-bucket
- Where finished, viewable videos livevideo-processing-function
- Lambda that handles new uploadstranscoding-job-queue
- MediaConvert queue for processingvideo-delivery-network
- CloudFront distributionvideo-metadata-table
- DynamoDB tablesearch-indexing-function
- Lambda that updates the search indexvideo-search-service
- OpenSearch domainuser-pool
- Cognito user management
The whole system flows like this:
[User Upload] → [raw-video-bucket] → [Event] → [video-processing-function] → [transcoding-job-queue]
↓
[User Viewing] ← [video-delivery-network] ← [processed-video-bucket] ← [Transcoded Videos]
Werner Vogels, Amazon’s CTO, describes this pattern as “choreography” where “each component reacts to events instead of being told what to do.” I love this approach because it creates a system that’s both simple and powerful.
Deepen your understanding in Building Pokémon GO on AWS: A Complete System Design Guide
The Upload and Processing Flow
Let me walk you through what happens when someone uploads a video:
User Uploads a Video
When Maria uploads her vacation video, our application gets a special pre-signed URL from AWS and uploads directly to our
raw-video-bucket
. This is efficient because the video doesn’t need to pass through our servers.S3 Triggers aws-lambda/">Lambda
As soon as the video finishes uploading, S3 automatically sends an event to our
video-processing-function
. This happens because we set up event notifications on our bucket that say “when a new object arrives, tell aws-lambda/">Lambda about it.”aws-lambda/">Lambda Processes the Video
Our Lambda function wakes up and receives information about Maria’s video - where it’s stored, its size, when it was uploaded. The function then:
- Creates a record in our metadata table saying “processing started”
- Analyzes the video to determine the best processing settings
- Creates a job specification for MediaConvert with instructions like “create three versions: 480p, 720p, and 1080p”
- Sends this job to MediaConvert’s
transcoding-job-queue
MediaConvert Does the Heavy Lifting
MediaConvert processes the video according to our specifications. It’s specially designed for video work and handles all the complex encoding operations. When it’s done, it places the processed videos in our
processed-video-bucket
.Completion Handling
When MediaConvert finishes, it sends a completion event. Another Lambda function catches this event and:
- Updates our metadata table to show the video is ready
- Adds information like video duration, thumbnail URLs, and available resolutions
- Makes the video searchable in our search service
Video Delivery
Now when someone wants to watch Maria’s vacation video, our application:
- Checks the metadata table to find available formats
- Selects the appropriate resolution based on the viewer’s device
- Delivers the video through CloudFront for fast, global access
The beauty of this design is that everything happens automatically once the video is uploaded. As Peter Sbarski notes in his book “Serverless Architectures on AWS,” this event-driven approach “creates systems that respond to changes as they happen.”
Explore this further in Top 10 Linux Automation Tools to Supercharge Your Workflow in 2025
Making Videos Searchable
A video platform isn’t much use if people can’t find the content they want! Here’s how we make videos searchable:
Metadata Collection
When Maria uploads her vacation video, she also adds a title (“Summer in Greece”), description (“Two amazing weeks island hopping”), and tags (“travel”, “beach”, “summer”).
Initial Database Entry
Our
video-processing-function
creates an entry in thevideo-metadata-table
with:- Video ID (a unique identifier)
- User ID (Maria’s account)
- Title, description, and tags
- Upload time
- Status (“PROCESSING”)
DynamoDB to Lambda to Search
We’ve configured DynamoDB to send changes to our
search-indexing-function
. When a record changes:- Lambda receives the change notification
- If the video is ready (status = “READY”), it formats the data for search
- It updates the
video-search-service
with the searchable content
Completion Updates
When MediaConvert finishes processing Maria’s video:
- The metadata record is updated with status “READY”
- Technical details are added (duration, formats, etc.)
- This change triggers the search indexing process
Search Experience
Now when someone searches for “Greece vacation”:
- Our search service finds Maria’s video based on title and description
- Results include thumbnails and basic details
- Users can filter by duration, upload date, and other attributes
This approach creates a seamless search experience similar to YouTube, where content becomes findable as soon as it’s ready for viewing.
Discover related concepts in 31 Reasons Why DevOps Are Better Lovers (Modern Psychology)
Making it Fast: Performance Optimization
Video processing can be slow, but I’ve learned several tricks to speed things up:
Right-Size Your Lambda
Our
video-processing-function
uses 3008MB of memory. This may seem like a lot, but with Lambda, more memory also means more CPU power. Chris Munns from AWS taught me that “increasing memory can actually reduce costs because functions finish faster.”Smart Video Analysis
Not all videos are created equal. Our Lambda quickly analyzes each upload to determine:
- Is it high motion (sports) or mostly static (lecture)?
- What’s the input resolution and quality?
- How long is the content?
Then it customizes the MediaConvert job accordingly.
Accelerated Transcoding
For most videos, we use MediaConvert’s accelerated transcoding, which can be up to 3x faster. It costs a bit more but dramatically improves the user experience - most people don’t want to wait hours to share their videos.
Parallel Processing
For longer videos, our system creates multiple smaller transcoding jobs that can run in parallel. This approach, recommended in the AWS Media blog, can turn a 2-hour processing job into 30 minutes.
Performance Monitoring
We track processing time for different video types and continually refine our settings. Our current performance benchmarks:
- 1-minute mobile video: ~45 seconds end-to-end
- 10-minute HD video: ~5 minutes
- 1-hour presentation: ~15 minutes
These optimizations mean Maria’s vacation video is ready to share much faster, creating a better user experience.
Uncover more details in Mastering CPU Cores: Strategies for Monitoring and Optimization
Keeping Videos Safe: Security Implementation
Security is critical for a video platform. Here’s how we protect content:
Secure Buckets
Our S3 buckets are locked down tight:
raw-video-bucket
only allows authenticated uploads- All data is encrypted at rest using server-side encryption
- Public access is completely blocked
Precise Permissions
Our Lambda functions have exactly the permissions they need and nothing more:
video-processing-function
can read from raw bucket, submit MediaConvert jobs, and write metadatasearch-indexing-function
can only read from DynamoDB and update the search service
User Management
Cognito’s
user-pool
handles authentication with:- Password policies requiring strong credentials
- Optional multi-factor authentication for sensitive operations
- Temporary credentials for uploads that expire after 15 minutes
Content Validation
Before processing, we validate uploads:
- Verify file is actually a video
- Check for maximum size limits
- Scan for potential security issues
Access Controls
Videos can be marked as:
- Public (anyone can view)
- Private (only the owner)
- Shared (specific users or groups)
Our system enforces these permissions at the application and CDN level.
These security measures follow AWS best practices outlined in their Well-Architected Framework and give users confidence their content is protected.
Journey deeper into this topic with A Complete Guide to AWS Security Features: Protecting Your Cloud Infrastructure
Watching Everything: Monitoring and Alerting
Good monitoring is like having a security camera system for your application. Here’s our approach:
Comprehensive Dashboard
We created a CloudWatch dashboard that shows:
- Upload counts by hour/day
- Processing success rates
- Average processing times
- Error counts and types
This gives us a quick overview of system health.
Smart Alerting
Not all issues are equal, so our alerts are tiered:
- Critical: Processing failures above 5% or system outages
- Warning: Performance degradation or capacity concerns
- Info: Unusual patterns worth investigating
End-to-End Tracking
We track videos through their entire journey:
- Upload success/failure
- Processing start/completion
- Delivery statistics
If a video enters our system but doesn’t complete processing within expected timeframes, we investigate automatically.
User Experience Metrics
Beyond just technical metrics, we track:
- Video loading times for viewers
- Buffering frequency
- Search response times
These affect how users perceive our platform.
Yan Cui, a serverless expert, says “good observability is essential for serverless applications.” I’ve found this to be absolutely true - you can’t improve what you can’t measure.
Enrich your learning with Mastering CPU Cores: Strategies for Monitoring and Optimization
Putting It All Together: The Complete System
The complete YouTube-like system combines all these components into a seamless whole. Here’s how it all fits together:
┌─── [search-indexing-function] ─── [video-search-service]
│
[User] ─── [Upload] ─── [raw-video-bucket] ─── [video-processing-function] ─── [transcoding-job-queue]
│ │
│ │
└─── [video-metadata-table] ◄────┘
│
│
[User] ◄─── [video-delivery-network] ◄─── [processed-video-bucket] ◄─── [MediaConvert]
The beauty of this design is its simplicity. Each component has a clear purpose and connects directly to the next. AWS handles all the infrastructure, scaling, and reliability concerns automatically.
When building your own YouTube-like system, I recommend starting with this approach because:
- It’s straightforward to implement - You can build a prototype in days
- It scales automatically - From 10 videos to 10 million
- It’s cost-effective - You only pay for what you use
- It requires minimal maintenance - No servers to patch or scale
James Hamilton from AWS puts it perfectly: “Focus on what makes your application special, and let the cloud handle the rest.”
Gain comprehensive insights from Building Pokémon GO on AWS: A Complete System Design Guide
Getting Started: Your Next Steps
Ready to build your own YouTube-like platform? Here’s how to get started:
Set Up Your AWS Account
If you don’t already have one, create an AWS account and familiarize yourself with the AWS console.
Create Your Resources
Begin by creating:
- S3 buckets for raw and processed videos
- A DynamoDB table for metadata
- A basic Lambda function for processing
Configure Event Triggers
Set up S3 event notifications to trigger your Lambda function when videos are uploaded.
Build a Simple Frontend
Create a basic web application that allows users to:
- Upload videos
- View their videos after processing
- Search for content
Iterate and Improve
Start simple and add features as you go:
- Multiple resolution support
- Enhanced search
- User profiles and subscriptions
The AWS Serverless Workshop provides excellent hands-on tutorials that follow a similar pattern to what we’ve discussed.
Building a YouTube-like system used to require dozens of servers, complex orchestration, and big budgets. Now, with this straightforward approach using AWS Lambda and S3, you can create a scalable, efficient video platform with minimal infrastructure and maintenance. The event-driven pattern makes everything simpler while giving your users a smooth, responsive experience.
I’m still amazed at how easy this approach makes what used to be an incredibly complex task. Now go build something awesome!
Similar Articles
Related Content
More from cloud
Master the Linux ls command with this comprehensive guide covering basic and advanced options, …
Master the Linux ls command with our task-oriented approach covering everyday file management …
You Might Also Like
A deep dive into the world of software architecture, examining the pros and cons of Top-Down and …
Learn how to build a streamlined serverless social network using core AWS services like Cognito, …
Discover how Lambda website integration can transform your static sites into dynamic web …
Knowledge Quiz
Test your general knowledge with this quick quiz!
The quiz consists of 5 multiple-choice questions.
Take as much time as you need.
Your score will be shown at the end.
Question 1 of 1
Quiz Complete!
Your score: 0 out of 1
Loading next question...
Contents
- Building a YouTube-like System: The Simple Approach
- System Overview: The Building Blocks
- The Upload and Processing Flow
- Making Videos Searchable
- Making it Fast: Performance Optimization
- Keeping Videos Safe: Security Implementation
- Watching Everything: Monitoring and Alerting
- Putting It All Together: The Complete System
- Getting Started: Your Next Steps