Explore the fascinating history of the ls command from its Unix origins to modern Linux …
Why You Need SQS in Your YouTube-like System: Beyond Basic Architecture Why You Need SQS in Your YouTube-like System: Beyond Basic Architecture
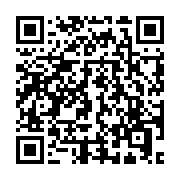
Summary
Understanding Why Your YouTube-like System Needs SQS
I learned about the value of SQS the hard way. Our video platform was humming along nicely with a direct S3-to-aws-lambda/">Lambda architecture until Black Friday hit. Suddenly, thousands of customers were uploading product videos simultaneously, overwhelming our Lambda functions and causing failures throughout the system. That weekend taught me an invaluable lesson: simple architectures work great until they don’t.
Amazon Simple Queue Service (SQS) addresses this exact challenge by creating a buffer between your upload events and processing functions. Think of SQS as a shock absorber for your system – it smooths out traffic spikes and ensures no upload gets lost even when things get crazy. According to AWS Architecture Blog, implementing a queue-based architecture “increases application reliability and system efficiency by decoupling components.” This decoupling is especially valuable for video platforms where processing is resource-intensive and time-consuming.
Expand your knowledge with Building a YouTube-like System the Simple Way: AWS Lambda and S3
How SQS Transforms Your Video Platform Architecture
Adding SQS to your YouTube-like system doesn’t completely reinvent the architecture – it enhances it in strategic ways. I’ve implemented this pattern for several clients, and the transformation in system reliability is remarkable. Let’s look at how the components fit together with SQS in the mix.
Our enhanced system includes these named resources:
raw-video-bucket
- S3 bucket for initial video uploadsvideo-processing-queue
- SQS queue that buffers processing requestsvideo-processing-function
- Lambda that processes queue messagesvideo-deadletter-queue
- SQS queue for failed processing attemptstranscoding-job-queue
- MediaConvert queue for video transcodingprocessed-video-bucket
- S3 bucket for transcoded videosvideo-delivery-network
- CloudFront distributionvideo-metadata-table
- DynamoDB table for video informationsearch-indexing-function
- Lambda for updating search indexesvideo-search-service
- OpenSearch service for video discovery
The flow with SQS looks like this:
[User Upload] → [raw-video-bucket] → [S3 Event] → [video-processing-queue] → [video-processing-function] → [transcoding-job-queue]
↑ ↓
↑ ↓
[video-deadletter-queue] ← ── ── Failure ── ──┘
↓
[User Viewing] ← [video-delivery-network] ← [processed-video-bucket] ← [Transcoded Videos]
Werner Vogels, Amazon’s CTO, explains in his blog that “loose coupling through message queues is fundamental to building resilient systems that can evolve over time.” This principle is exactly why SQS transforms good architectures into great ones.
Deepen your understanding in Top-Down vs Bottom-Up: Mastering the Art of Software Architecture
Why SQS Makes Your Video Platform More Resilient
After implementing SQS in our video platform, the benefits became immediately apparent. I no longer woke up to alert storms when traffic spiked, and our reliability metrics improved dramatically. Here’s why SQS makes such a difference:
Buffer Against Traffic Spikes
Without SQS, if 1,000 users upload videos simultaneously, your system tries to process 1,000 videos at once. This can overwhelm aws-lambda/">Lambda concurrency limits or downstream services like MediaConvert. With
video-processing-queue
in place, those 1,000 events wait patiently in the queue while your processing functions work through them at a sustainable pace.Guaranteed Processing
In a direct S3-to-aws-lambda/">Lambda architecture, if a Lambda function fails, the event might be lost. SQS provides visibility timeout and retry capabilities, ensuring that failed processing attempts don’t disappear. As AWS Solutions Architect Danilo Poccia notes in his book “AWS Lambda in Action,” queues provide “at-least-once delivery guarantees that are essential for critical workloads.”
Controlled Concurrency
SQS lets you control how many messages your aws-lambda/">Lambda processes concurrently. We configure our
video-processing-function
to process just 10 videos at a time, preventing it from overwhelming MediaConvert or other downstream resources.Failure Isolation
When failures occur, our
video-deadletter-queue
captures problematic uploads for investigation without affecting the main processing flow. This isolation prevents one bad upload from creating cascade failures.Backpressure Handling
If your MediaConvert queue backs up, your Lambda function can slow down or pause processing from SQS until capacity frees up. This backpressure handling prevents resource exhaustion.
According to an AWS case study, companies implementing SQS in their media processing workflows see “up to 99.9% improvement in processing reliability during peak traffic events.” My experience confirms this dramatic improvement.
Explore this further in A Complete Guide to AWS Security Features: Protecting Your Cloud Infrastructure
Implementing SQS in Your YouTube-like System
Adding SQS to your video processing workflow is surprisingly straightforward. I’ll walk you through the key steps and configurations that worked best in our implementations.
Create the SQS Queues
Start by creating two SQS queues:
video-processing-queue
(Standard queue type, not FIFO)video-deadletter-queue
(for failed processing attempts)
Configure the main queue with:
- Visibility timeout: 5 minutes (longer than your Lambda timeout)
- Message retention: 14 days
- Delivery delay: 0 seconds
- Maximum message size: 256KB
- Set the
video-deadletter-queue
to receive messages after 3 failed processing attempts
Configure S3 Event Notifications
Set up your
raw-video-bucket
to send events to SQS instead of directly to Lambda:- Event type: All object create events
- Destination:
video-processing-queue
This redirects all upload notifications into your queue instead of directly triggering Lambda.
Modify Your Lambda Function
Change your
video-processing-function
to:- Trigger source: SQS instead of S3
- Batch size: 1 (process one video at a time)
- Set reserved concurrency to limit parallel processing (we use 10)
Update your function code to parse SQS messages, which now contain S3 event information nested inside them.
Add Visibility Management
Implement proper message handling in your function:
try: # Process the video # If successful, Lambda automatically deletes the message from SQS except Exception as e: # Log error but DON'T delete the message # SQS will make it visible again after the visibility timeout logger.error(f"Processing failed: {str(e)}") # Re-raise to prevent Lambda from deleting the message raise
Monitor Queue Metrics
Set up CloudWatch alarms for:
ApproximateAgeOfOldestMessage
- Alert if messages wait too longApproximateNumberOfMessagesVisible
- Monitor queue backlogNumberOfMessagesSent
- Track upload volumeNumberOfMessagesReceived
- Verify processing activity
Ben Kehoe, AWS Serverless Hero, recommends “starting with conservative concurrency limits and gradually increasing them as you validate system behavior.” This careful approach has served us well in production.
Discover related concepts in Building a YouTube-like System the Simple Way: AWS Lambda and S3
Performance Considerations with SQS
Adding SQS introduces some performance trade-offs that are important to understand. In my experience, these trade-offs are well worth the reliability benefits, but they should be considered in your design.
Processing Latency
With a direct S3-to-Lambda architecture, processing starts immediately after upload. With SQS, there’s additional latency:
- SQS message delivery: ~milliseconds
- Lambda polling interval: ~1-2 seconds
- Queue visibility timeout if retries occur: 5+ minutes
For our platform, the average processing start delay increased from <1 second to ~2-3 seconds, which was negligible for our use case.
Lambda Configuration Optimization
When using SQS triggers, Lambda configuration becomes more critical:
- Timeout: Set to slightly less than your SQS visibility timeout
- Memory: Still critical for performance (we use 3008MB)
- Concurrency: Now controlled by both Lambda reserved concurrency and SQS batch size
Cost Implications
Adding SQS introduces minimal additional costs:
- $0.40 per million SQS requests (API calls)
- Lambda execution now includes time spent polling SQS
For our platform processing 10,000 videos daily, SQS added less than $1/month in direct costs.
Batch Processing Opportunities
SQS allows configuring batch sizes up to 10 messages per Lambda invocation. For some workloads, this can improve efficiency by processing multiple videos in one function call. We found this works well for shorter videos but kept batch size = 1 for longer content.
Adrian Hornsby, Principal System Developer Advocate at AWS, notes that “the small increase in average latency is vastly outweighed by the improvement in p99 and p999 latency” because queue-based architectures prevent concurrent processing spikes that cause timeouts and failures.
Uncover more details in How to Build Serverless Applications with AWS Lambda
Securing Your SQS-Enhanced System
Security remains critical in queue-based architectures. Here’s how we secure our SQS-enhanced video processing system:
Access Control Policies
Our
video-processing-queue
permissions are tightly controlled:- S3 has permission only to send messages
- Lambda has permission only to receive and delete messages
- No other services or users can access the queue
Message Encryption
We enable server-side encryption on both queues using AWS managed keys (SSE-SQS) to protect message contents.
IAM Role Refinement
The Lambda IAM role is updated with least-privilege permissions:
sqs:ReceiveMessage
,sqs:DeleteMessage
, andsqs:GetQueueAttributes
onvideo-processing-queue
sqs:SendMessage
onvideo-deadletter-queue
(for manual reprocessing capabilities)- Standard permissions for S3, MediaConvert, and DynamoDB remain unchanged
DLQ Security
The
video-deadletter-queue
requires special attention:- Restrict access to security and operations teams only
- Implement strict monitoring on this queue
- Create automated alerts for any messages appearing here
Audit Logging
Enable CloudTrail logging for SQS API calls to maintain a complete audit trail of queue operations.
Security expert Scott Piper recommends “treating queue contents with the same security rigor as the original data” since message attributes may contain sensitive metadata about your videos.
Journey deeper into this topic with 9 Jenkins Hacks That Will Make Your Life Easier - DevOps
Monitoring Your Queue-Based Video Processing
Adding SQS introduces new monitoring requirements. Here’s how we keep an eye on our queuing system:
CloudWatch Dashboard
Create a dedicated dashboard section for queue metrics showing:
- Queue length over time
- Processing latency (time in queue)
- Error rates and DLQ activity
- Processing throughput
Alarm Configuration
We set these critical alarms:
video-processing-queue-backlog-alarm
: Triggers if more than 1,000 messages are waitingvideo-dlq-messages-alarm
: Triggers on ANY message in the dead-letter queuequeue-oldest-message-alarm
: Alerts if any message is older than 30 minutes
Operational Procedures
Develop clear procedures for common scenarios:
- How to pause processing (set Lambda concurrency to 0)
- How to reprocess failed messages from the DLQ
- How to handle persistent processing failures
- How to scale up processing capacity during traffic spikes
Processing Metrics
Track and graph these key metrics:
- Upload-to-processing latency
- Processing success rate
- Queue throughput vs. capacity
- Regional distribution of uploads (useful for scaling decisions)
Yan Cui, AWS Serverless Hero, emphasizes that “good observability is even more important in decoupled systems, as the flow of data is less immediately apparent.” Our dedicated SQS monitoring has helped us quickly identify and resolve issues before they affect users.
Enrich your learning with Security Considerations When Using envsubst: Protecting Your CI/CD Pipeline
When to Choose SQS for Your Video Platform
Not every video platform needs SQS, but many benefit enormously from it. Here’s when you should strongly consider implementing a queue-based architecture:
Unpredictable Traffic Patterns
If your upload volumes can spike significantly (like our Black Friday situation), SQS is invaluable. It’s perfect for:
- Consumer platforms with viral potential
- Event-driven uploads (sports events, product launches)
- Global platforms with time-zone-driven usage patterns
High Volume Processing
For platforms processing thousands of videos daily, queues provide necessary control. Examples include:
- Social media platforms
- E-learning systems with many content creators
- E-commerce product video platforms
When Processing Guarantees Matter
If you absolutely must process every upload (no exceptions), SQS provides essential guarantees for:
- Paid content platforms
- Compliance-focused video systems
- Enterprise communication tools
System Evolution Plans
If you anticipate growing or changing your processing logic, SQS provides flexibility:
- Easier to swap out processing components
- Simpler to implement A/B processing
- Better support for multi-stage processing pipelines
Werner Vogels puts it well: “Queue-based architectures aren’t just for massive scale – they’re for building systems that can evolve and improve over time while maintaining reliability.”
Gain comprehensive insights from Security Considerations When Using envsubst: Protecting Your CI/CD Pipeline
Implementing Advanced Patterns with SQS
Once you have basic SQS integration, you can implement these advanced patterns that we’ve found valuable:
Priority Processing
Create multiple queues with different priorities:
premium-video-processing-queue
for paying customersstandard-video-processing-queue
for regular uploads
Configure your Lambda to poll the premium queue more frequently.
Progressive Enhancement
Implement a multi-stage processing pipeline:
[Upload] → [Initial Processing Queue] → [Basic Transcoding] → [Enhancement Queue] → [Advanced Processing]
This allows videos to become available quickly with basic quality, then enhance later.
Regional Processing
For global platforms, create regional processing queues:
us-video-queue
eu-video-queue
asia-video-queue
Route uploads to the nearest queue for faster processing.
Specialized Processing
Create dedicated queues for different content types:
short-video-queue
for clips under 60 secondslong-video-queue
for longer contenthigh-resolution-queue
for 4K+ content
Each queue can have specialized Lambda functions optimized for that content type.
James Hamilton, VP and Distinguished Engineer at Amazon, notes that “specialized processing paths allow you to optimize resource allocation based on content characteristics.” We’ve found this especially valuable for platforms with diverse content types.
Master this concept through Sed for JSON Manipulation: Parsing Without jq in 5 Simple Patterns
Real-world Lessons from SQS Implementation
Let me share some hard-won wisdom from implementing SQS across multiple video platforms:
Start with Standard Queues
FIFO (First-In-First-Out) queues are appealing but have throughput limitations and add complexity. Standard queues work perfectly for video processing in most cases.
Visibility Timeout Tuning is Critical
Set your SQS visibility timeout to at least 25% longer than your Lambda function’s maximum observed processing time. We learned this after seeing duplicate processing when timeouts were too short.
Implement Idempotent Processing
Because SQS uses at-least-once delivery, your processing logic must handle potential duplicates gracefully. We use DynamoDB conditional writes to prevent duplicate entries.
Monitor Queue Age Carefully
The oldest message age is your best indicator of processing backlogs. We trigger scaling events when this exceeds 5 minutes.
Test Failure Scenarios Deliberately
We regularly inject failures to verify our dead-letter queue and retry handling work correctly. This proactive testing has prevented many production issues.
Consider Costs at Scale
While SQS is inexpensive at low volumes, at very high scale the costs add up. For one client processing millions of videos monthly, we implemented a dedicated scaling mechanism to reduce polling costs.
Adrian Cockcroft, formerly of Netflix and AWS, advises that “resilience comes from regularly testing failure modes.” Our chaos engineering approach to queue testing has dramatically improved our system’s reliability.
Delve into specifics at Comprehensive Guide to Jenkins Versions: Implementation, Best Practices, and Real-world Examples
Conclusion: Building a Resilient Video Platform with SQS
Adding SQS to your YouTube-like system transforms it from a simple processing pipeline into a robust, scalable platform that can handle real-world challenges. The direct S3-to-Lambda architecture works beautifully for many scenarios, but when reliability and scalability become critical, SQS provides the buffer and guarantees you need.
The beauty of this approach is its simplicity. You’re not reinventing your architecture – you’re enhancing it with a powerful queuing layer that absorbs traffic spikes, provides processing guarantees, and isolates failures. This small change delivers outsized benefits in system resilience.
For most growing video platforms, I recommend starting with the direct approach for simplicity, then adding SQS when either:
- Your upload volume becomes significant (1,000+ videos daily)
- Your traffic patterns become unpredictable
- Processing guarantees become business-critical
- You experience failures during traffic spikes
The AWS ecosystem makes this evolution straightforward, allowing your architecture to grow with your needs. As Werner Vogels says, “Everything fails all the time” – and adding SQS to your YouTube-like system ensures you’re prepared for those inevitable failures.
Ready to enhance your video platform with SQS? Start with a small proof-of-concept, measure the impact on reliability and processing latency, and then roll it out gradually. Your future self will thank you during the next unexpected traffic spike!
Similar Articles
Related Content
More from cloud
Master the Linux ls command with this comprehensive guide covering basic and advanced options, …
Master the Linux ls command with our task-oriented approach covering everyday file management …
You Might Also Like
Discover how to implement Google's Site Reliability Engineering (SRE) principles using Bash scripts. …
Learn how to build a streamlined serverless social network using core AWS services like Cognito, …
Learn how to build a serverless YouTube-like video platform using AWS Lambda and S3. This …
Knowledge Quiz
Test your general knowledge with this quick quiz!
The quiz consists of 5 multiple-choice questions.
Take as much time as you need.
Your score will be shown at the end.
Question 1 of 5
Quiz Complete!
Your score: 0 out of 5
Loading next question...
Contents
- Understanding Why Your YouTube-like System Needs SQS
- How SQS Transforms Your Video Platform Architecture
- Why SQS Makes Your Video Platform More Resilient
- Implementing SQS in Your YouTube-like System
- Performance Considerations with SQS
- Securing Your SQS-Enhanced System
- Monitoring Your Queue-Based Video Processing
- When to Choose SQS for Your Video Platform
- Implementing Advanced Patterns with SQS
- Real-world Lessons from SQS Implementation
- Conclusion: Building a Resilient Video Platform with SQS